Very often you may need to handle POST request data in Django. In this article, we will look at how to retrieve POST request data in Django.
How to Retrieve POST Request Data in Django
Here are the steps to retrieve POST request data in Django. Let us say you have the following HTML form on your web page.
<form method="post" action="/test"> <input type="text" name="username" /> <input type="text" name="email" /> <input type="submit" value="Submit" /> </form>
When a user fills out the above form and clicks Submit button, Django will receive a POST request object from the client browser, containing the form information.
To capture the POST data, you will need to create a view. We will look at both class-based and classless views for this purpose.
Using Class-based views
Here is an example of a class-based view to capture POST request data in Django. First, you will need to define the appropriate URL configuration that links URL to the view handler, as shown. Replace myapp with your app name, and MyView with your class-based view’s name
# urls.py from django.urls import path from myapp.views import MyView urlpatterns = [ path('test/', MyView.as_view()), ]
Next, you will need to define a class-based view as shown below.
from django.http import HttpResponse from django.views import View class MyView(View): def post(self, request): email = request.POST.get("email") username = request.POST.get("username") return HttpResponse('email:'+email+'\n username:'+username')
Let us look at the above lines in detail. We have created class-based view MyView. In its post view, we access form fields using request.POST.get() function. In each function call we have used the form field’s name attribute present in HTML page, to fetch that field’s value. For example, since the email form field’s name attribute is ’email’ in the HTML page, you can access it as request.POST.get(’email’).
Without Using Class-based Views
If you don’t use class-based views, you can access the POST data using classless views, as shown below. In this case, you can easily define the URL configuration as shown below.
# urls.py from django.conf.urls.defaults import * from apps.dashboards.views import * urlpatterns = patterns('', url(r'test$',my_view,name='test'),
Next, you will need to define the following view to handle POST data.
from django.http import HttpResponse def my_view(request): if request.method == 'POST': email = request.POST.get("email") username = request.POST.get("username") return HttpResponse('email:'+email+'\n username:'+username')
In this view, first we check the request method. If it is POST only then we call request.POST to read form data. If you call request.POST for GET requests, you will get an error.
Further, we access form fields using request.POST.get() function, just as we did with class-based view. In each function call we have used the form field’s name attribute present in HTML page, to fetch that field’s value. For example, since the email form field’s name attribute is ‘username’ in the HTML page, you can access it as request.POST.get(‘username’).
In this article, we have seen how to retrieve POST data in Django. Depending on your Django version and preference, you can choose to use class-based view or classless view.
Also read:
How to Capture URL Parameters in Django
How to Lookup Dictionary Value in Django Template
How to Loop Through List in Django Template
How to Run Python Script in Django Shell
How to Disable CSRF Validation in Django
Related posts:
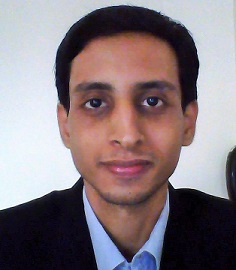
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.