By default, Django offers CSRF protection to secure your website from cross site requests. However, in some cases you may need to disable CSRF protection to be able to accept and process requests from other website domains. In this article, we will look at how to disable CSRF validation in Django view.
What is CSRF Validation
Cross Site Request Forgery (CSRF) is an attack whereby a malicious code such as link, form, javascript, etc on another website sends a request to your server using the credentials of a logged in user on your website, when he/she also visit the other website. Django provides a middleware and template tag to prevent CSRF attacks by automatically checking for the host & domain names of requests, as well as setting server-based exchange tokens for forms. It also provides cookie-based validation for this purpose. Django CSRF middleware is enabled by default, meaning every Django view is protected from CSRF unless you manually disable it.
How to Disable CSRF validation in Django View
Here are the steps to disable CSRF validation in Django view. There are a couple of ways to disable CSRF protection in Django.
1. Using @csrf_exempt decorator
CSRF protection is enabled by default for all Django views. If you want to disable CSRF protection for one or more views, but not all views then add the following line in that view’s views.py file
from django.views.decorators.csrf import csrf_exempt
The is will import the @csrf_exempt decorator that allows you to easily disable CSRF validation for specific views. Just place @csrf_exempt decorator immediately above the view for which you do not want CSRF protection.
@csrf_exempt def my_view(request): return HttpResponse('Hello world')
So your views.py file will look like
from django.views.decorators.csrf import csrf_exempt
...
@csrf_exempt
def my_view(request):
return HttpResponse('Hello world')
2. Disable CSRF middleware
If you want to disable CSRF protection for all views, simply comment (by adding # at its beginning) or delete CSRF middleware from settings.py file.
MIDDLEWARE = [
...
#'django.middleware.csrf.CsrfViewMiddleware',
...
]
However, please note, CSRF protection is a very good security measure and you must not disable it for all views on your site, unless it is absolutely necessary. The recommended way to disable CSRF validation is to use the @csrf_exempt decorator only for views where you don’t want it, as shown above.
Also read:
How to Enable CORS in Django Project
How to Combine Querysets in Django
How to Fix NoReverseMatch Error in Django
How to Convert PDF to Image/JPG in Linux
How to Redirect Apache with Query String
Related posts:
Django Get Unique Values From Queryset
How to Retrieve POST Request Data in Django
How to Get Classname of Instance in Python
How to Loop Through List in Django Template
How to Terminate Python Subprocess
How to Uninstall Django in Ubuntu
How to Use Sleep Function in Python
Plot Graph from CSV Data Using Python Matplotlib
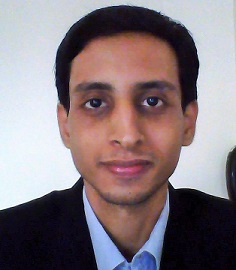
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.