Django querysets allow you to query objects and retrieve values. They are equivalent to running database queries. While working with Django querysets you may need to retrieve distinct values from objects. In this article, we will learn how to get unique values in queryset.
Django Get Unique Values From Queryset
Django provides a distinct() function to easily get distinct values of queryset result. Let us say you have an object Sales(user_id, product_id, order_id, date, amount)
Let us say you want to retrieve all unique user_id values from your sales table, then here is the query for it.
distinct_users = Sales.objects.all().values_list('user_id', flat=True).distinct()
If you want to get distinct objects, instead of values, then remove flat=True from the above query, and use values() instead of values_list().
distinct_users = Sales.objects.all().values('user_id').distinct()
In the above code, we add distinct() at the end of queryset to get distinct values. Django will first retrieve list of all user_id values, and then get distinct values of out of them.
If you want to get a count of the above distinct values, you can chain count() function after distinct() function.
count_distinct_users = Sales.objects.all().values_list('user_id', flat=True).distinct().count()
If you want to filter the data and get distinct values out of the filtered result, then you can use filter() function instead of all(), before distinct(), as shown below. Here is the query to get distinct user_id for sales rows where product_id=12345
distinct_users = Sales.objects.filter(product_id=12345).values('user_id').distinct()
In this short article, we have learnt different ways to get distinct values from queryset in Django.
Also read:
How to Create RPM from Python Module
What is NoReverseMatch Error and How to Fix It
How to Create RPM for Shell Script
How to Enable Email Alerts in Keepalived
How to Use NMAP in Kali Linux
Related posts:
How to Create Cartesian Product of Two Lists in Python
How to Use Sleep Function in Python
How to Schedule Task in Python
How to Compare Dictionary in Python
How to Find Django Install Location
How to Delete Objects in Django
How to Convert Columns into Rows in Pandas
How to Get Unique Values from Array in JavaScript
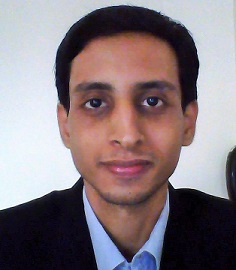
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.