Django is a popular web framework that allows you to quickly build websites & applications. In many cases, you may need to delete objects in Django. In this article, we will learn how to delete objects in Django.
How to Delete Objects in Django
Here are the different ways to delete objects in Django. Let us say you have a model Post (post_id, author_id, post_data, published_date) where each row contains data about each blog post.
Delete All Objects
The general syntax to delete all objects in a model is
Model.objects.all().delete()
Here is the command to delete all objects in Post model.
Post.objects.all().delete()
If you need to delete only a specific subset of objects, you can use filter function to select those rows first, and then use delete() function to delete them. Here is an example to delete all posts with future published date value.
Post.objects.filter(published_date__gt=datetime.now()).delete()
Please note, if there are other models with foreign keys pointing to Post model, then you may get an error during deletion, due to foreign key constraint.
Delete Related Objects
Let us say you have a Model Author(id, name) such that Post (post_id, author_id, post_data) has a foreign Key that points to Author id in Author model. Sometimes you may need to delete all posts of an author. Here is the command to delete all posts by author ‘John Doe’.
Post.objects.filter(author__name='John Doe').delete()
In the above case, Django will look up id from Author objects where author name =’John Doe’ and use it to filter the relevant posts in Post column. Then it will delete the selected posts.
The django querysets where we use filter() function to select multiple rows and use delete() function to delete them is also known as bulk deletion.
In this article, we have learnt how to delete objects in Django.
Also read:
How to Delete File or Folder in Python
How to Disable Django Error Emails
How to Add Minutes, Hours & Months in PostgreSQL
Find Files with Special Characters in Filename in Linux
Find Last Character in String in Javascript
Related posts:
How to Read File Line by Line Into Python List
Call Python Function by String Name
How to Check if String Matches Regular Expression
How to Convert Columns into Rows in Pandas
How to Lock File in Python
How to Use Sleep Function in Python
How to Fix NoReverseMatch Error in Django
How to Call C Function in Python
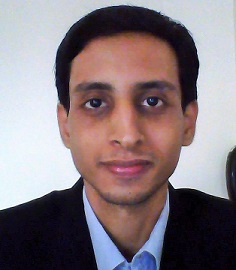
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.