Python is a powerful programming language that supports tons of functions and has a vast package library. But sometimes you may need to run a C function from within Python. This may happen if you are supporting legacy systems written in C that you want to call from your new code written in python. Since C is faster than python, it may also be needed if you want to call a speed critical program written in C, that you want to invoke from within python. In this article, we will learn how to call C function in Python.
How to Call C Function in Python
Here are the steps to call C function in Python. One of the key differences between python and C is that C runs compiled programs while python does not require program compilation, since it uses runtime compiler. So we will need to compile the written C code and create a library out of it, before you can call it in a python script. So we have assumed you already have a C-compiler installed on your system.
1. Write C Program
First we create a C Program in a file called function.c. It simply accepts an integer input and checks its value. If it is 0 it will do nothing. If it is a power of 2 then returns 1 else it returns 0.
int myFunction(int num) { if (num == 0) // if number is 0, do not perform any operation. return 0; else // if number is power of 2, return 1 else return 0 return ((num & (num - 1)) == 0 ? 1 : 0) ; }
2. Compile C Program
Next, we run the following command to compile C Program function.c.
$ cc -fPIC -shared -o libfun.so function.c
The above command will create a shared library with filename libfun.so.
3. Call C Function from Python
Create a blank python script.
$ vi function.py
Add the following lines to it, to import the above created libfun.so shared library into it. For this purpose, we will use the ctypes python module that allows you to call C function from within python code.
import ctypes NUM = 16 fun = ctypes.CDLL("libfun.so") fun.myFunction.argtypes = [ctypes.c_int] returnVale = fun.myFunction(NUM)
In the above code, we first import ctypes module. Then we load the share library libfun.so using ctypes.DLL() function and store it in an object fun. Once it is loaded, you can access your myFunction as fun.myFunction(). But C has strict type checking for function arguments, unlike python. So we specify the argument of python function call to by integer (ctypes.c_int). This will ensure type checking when you call C-function from within python.
Once all this is done, you can easily call C function as fun.myFunction() just as you call python function, store its return value in python variables and use it further as per your requirement.
In this article, we have learnt how to call C function in python.
The most important advantage of using C functions in python is its speed. Since C program are pre-compiled, they improve python program’s speed of execution. It is a great way to re-use existing C code, without actually having to migrate from C to python systems. So instead of rewriting all C code in python, you can simply call your existing C functions from python script, making it easier to migrate your application to python.
Also read:
How to Change Default Data Directory in MySQL
How to Run MySQL Query from Command Line
How to Restore MongoDB Dump in Windows & Linux
Script to Keep Your Computer Awake
How to Extract Database from MySQL dump file
Related posts:
How to Download Images in Python
How to Convert PDF to CSV in Python
How to Count Repeated Characters in String in Python
How to Merge PDF Files Using Python
How to Upgrade All Python Packages with Pip
What Is __name__ In Python
How to Extract Tables from PDF in Python
How to Remove HTML Tags from CSV File in Python
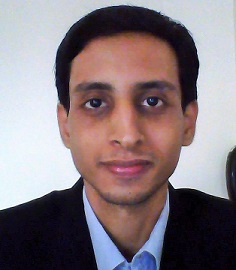
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.