While working with python code, you may have noticed __name__ appearing at different places of your code. Have you ever wondered what it means and how to use it? In this article, we will learn what is __name__ in python, when to use it and how to use it?
What Is __name__ In Python
In short, __name__ is basically a guard that protects your code from being invoked unintentionally. For example, if your script without __name__ in it is imported by a second script, then the second script will accidentally execute all lines of the first script during import, and will also the second script’s command line arguments. Similarly, if you have a guardless script in a class that you pickle, then pickling will trigger the guardless script.
Let us understand why this happens. When python executes script, its interpreter will automatically set __name__ variable. It is like a context variable. If we run ‘python foo.py’ command then __name__=’__main__’ when python runs code within foo.py file. In this case, it will execute all the source code in it.
Let us look at the following code foo.py
#foo.py file print("before functionA") def functionA(): print("Function A") print("before __name__ guard") if __name__ == '__main__': functionA() print("after __name__ guard")
In this case, when you run
$ python foo.py
__name__=’__main__’ when interpreter is running the code within foo.py. So in this case, it will also run the last but one line, where we call functionA(). This is important as you will see below. So you will see the following output.
before functionA before __name__ guard functionA after __name__ guard
Now let us say you create another file bar.py which imports foo.py
# file bar.py import foo print("bar")
Now let us run bar.py with the following command.
$ python bar.py
In this case, the python interpreter will set __name__=’foo’ and not ‘__main__’, when it imports this module. Now what happens is that when your foo.py module is imported python interpreter will start executing its code line by line. It will print ‘before functionA’, ‘before __name__ guard’, and ‘after __name__guard’. It will not execute the last but one line where we call functionA() because in this case, when python interpreter is executing the code of foo.py, the value of __name__ is ‘foo’ and not ‘__main__’ and therefore python skips the if block of code in foo.py. You will see the following output.
before functionA before __name__ guard after__name__ guard bar
But remember that __name__=’__main__’ before and after the import of foo.py, not during the import.
So in other words, __name__ guard can be used to prevent code from being executed, when a python is script is imported and not executed directly.
This is useful if you want to write a script whose code can be used by other scripts, but it can also be run on its own. It can also be used to create a python script that runs as a standalone script, but also provides helper functions and APIs for other modules. It can also be used to isolate unit tests without letting it run when the script is imported by other modules. You can also print the value of __name__ variable for debugging purposes, to understand where the error occurs. Please note, you can always have multiple __name__ checks if you want, though it doesn’t really help. You can manually change the value of __name__ if you want but you have to be careful as it can give unexpected output.
That’s it. In this article, we have learnt what is __name__, when to use it and how to use it. In short, when the python interpreter is executing the lines of main python script that is being run, __name__ will be __main__. When it is inside another module that is being imported, __name__ will be the name of that module.
Also read:
How to Find Non-ASCII Characters in MySQL
How to Use Reserved Word as Column in MySQL
How to Show Banned IP in Fail2ban
How to Unban IP in Fail2ban
NGINX Allow Local Network
Related posts:
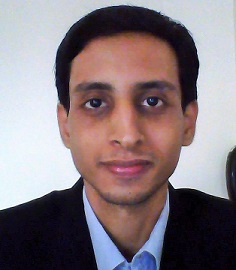
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.