Python List is a powerful data structure that allows you store large amount of diverse data types and process them quickly. It is often used by python developers in applications and websites. Sometimes you may need to count occurrence of list item in Python. There are several ways to do this. In this article, we will look at some of the simple ways to count occurrence of list item in Python.
How to Count Occurrence of List Item in Python
Let us say you have the following python list.
lst = [1,2,3,4,4,2,5,6,7]
1. Using for loop
You can simply loop through the list and update a counter variable to count the number of occurrences of a given list item. In this case, our function countX takes two arguments – list and element whose occurrence to find. We loop the list elements one by one and increment the counter variable count by one whenever we encounter the element.
# Python code to count the number of occurrences def countX(lst, x): count = 0 for ele in lst: if (ele == x): count = count + 1 return count print(countX(lst, 4))
You will get the following output.
2
2. Using Count() method
Every python list features a count() method t count number of occurrences of an item in the list. It takes the input as the element whose count you want to calculate, and returns the count.
# Python code to count the number of occurrences def countX(lst, x): return lst.count(x) print(countX(lst, 2))
You will get the following output.
2
3. Using Counter() method
The above methods return count of only single element. What if you want to count occurrences of all elements in list? For this purpose, you can use coutner() method. It returns a dictionary with occurrences of all elements as key-value pairs where key is the list item and value is the occurrence.
from collections import Counter d = Counter(lst) print(d)
You will see the following output.
{1:1,2:2,3:1,4:2,5:1,6:1,7:1}
In this article, we have learnt a few simple ways to count list item occurrences in Python.
Also read:
How to Restore Default Repositories in Ubuntu
How to Get Unique IP Address from Log File
How to Merge JS Objects
How to Restrict Internet Access for Programs in Linux
How to Check if String is Valid Number in JavaScript
Related posts:
Call Python Function by String Name
How to Get Field Value in Django Queryset
How to Comment in Python
How to Split File in Python
How to Create Cartesian Product of Two Lists in Python
How to Intersect Two Dictionaries in Python
How to Convert Columns into Rows in Pandas
How to Use Variable in Regex in Python
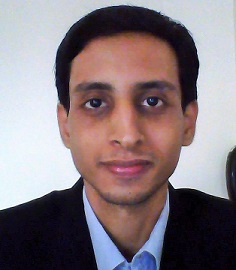
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.