Python allows you to store data as lists, dictionaries, or even list of dictionaries. Sometimes you may need to sort list of dictionaries by value in Python. In this article, we will learn how to do this kind of sorting.
How to Sort List of Dictionaries by Value in Python
Let us say you have the following list of dictionaries in Python.
lst = [{'name': 'Homer', 'age': 39}, {'name': 'Bart', 'age': 10}]
Let us say you want to sort it as shown below, based on name key.
lst = [{'name': 'Bart', 'age': 10}, {'name': 'Homer', 'age': 39}]
There are a couple of simple ways to sort this list of dictionaries based on values of name key.
The first method is using sorted() function and using key parameter. It returns a new sorted list
newlist = sorted(lst, key=lambda d: d['name'])
In the above function, key specifies the function of one argument used to get comparison key from each element in the list.
If you want to sort the dictionaries in descending order of values, you can use reverse=True argument.
newlist = sorted(lst, key=lambda d: d['name'], reverse=True)
Alternatively, you can also use operator.itemgetter instead of defining yourself.
from operator import itemgetter newlist = sorted(lst, key=itemgetter('name'))
Every list has a built-in sort() function that can be used to sort its elements. You can also use itemgetter within this function to sort list of dictionaries by value. Here is the command to sort the list by value of key name.
lst.sort(key=operator.itemgetter('name'))
In this article, we have learnt how to sort list of dictionaries by value in Python.
Also read:
How to Count Occurrence of List Item in Python
How to Restore Default Repositories in Ubuntu
How to Get Unique IP Address from Log Files
How to Merge JS Objects
How to Restrict Internet Access to Programs in Linux
Related posts:
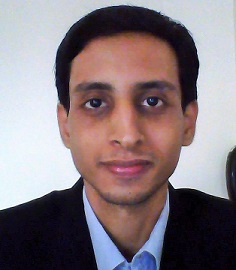
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.