Generally, python functions are called using their name as literals. But sometimes you may need to call a python function using string variable. In this article, we will learn how to call python functions using their names as strings.
Call Python Function by String Name
For example, let us say you have a function abc(), then here is how you typically call this function, by typing its name literally.
abc()
But sometimes, you may have a string variable with the function’s name as its value.
d="abc"
and you may want to call the function using this string variable.
If you use the following syntax, you will get an error.
d() OR "abc"()
So we will look at how to overcome this problem. There are different ways to invoke a function using string variable. We will look at the different use cases for this. First, if the function to be called is in the same module from where you are calling it, then you can use locals() or globals() to call the function by its string. locals() contains the local symbol table while globals() contains global symbol table. These symbols tables contain function names as well as their reference locations. So they can be used to call functions. Here are the different ways to call the function abc() using string “abc” or string variable d defined earlier.
locals()["abc"]() OR locals()[d]() OR globals()["abc"]() OR globals()[d]()
If your function abc() is inside another class E, then you need to modify your function call as shown below. Let us say you have class E with function abc().
class E: def __init__(self): pass def abc(self, arg): print('you called abc({})'.format(arg)) m = globals()['E']() func = getattr(m, 'abc') func('sample arg') # Sample, all on one line getattr(globals()['E'](), 'abc')('sample arg')
In the above code, we have created a class E with function abc() that accepts arguments and displays them. We use globals() to refer to the class E first. Then we use getattr() function to refer to function abc() within class E and store the reference in a variable func. Then using this reference, we call it and pass an argument ‘sample arg’ to it.
This approach can be used for calling functions from within modules also. Here is an example to import module foo and call function bar within this module using its string name.
module = __import__('foo') func = getattr(module, 'bar') func()
In this article, we have learnt several ways to call python function by string. You can use any of them as per your requirement.
Also read:
How to Save Git Username & Password
How to Get Classname of Instance in Python
How to Lock File in Python
How to Use Boolean Variables in Shell Script
How to Concatenate String Variables in Shell Script
Related posts:
How to List All Virtual Environments in Python
How to Read File Line by Line Into Python List
How to Run Python Script in Apache Web Server
How to Find Django Install Location
How to Reverse/Invert Dictionary Mapping in Python
How to Randomly Select Item from List in Python
How to Read Binary File in Python
What Is __name__ In Python
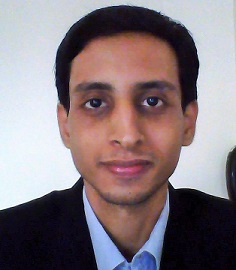
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.