Sometimes you may need to create python dictionary from string. Such strings also called as non-standard JSON, unless their quotes conform to JSON standard. In this article, we will learn how to convert string representation of dictionary into dictionary using python. There are several ways to do this. We will learn 3 simple ones for our purpose.
How to Create Python Dictionary from String
Let us say you have the following string representation of dictionary. Please note, our string has double quotes at the end, and single quotes around the key and value data.
s = "{'name' : 'jane', 'nickname' : 'kitty'}"
We want to convert the above string to following dictionary.
s={'name' : 'jane', 'nickname' : 'kitty'}
1. Using literal_eval
You can use literal_eval function available in ast library of python, as shown below.
>>> import ast >>> ast.literal_eval("{'name' : 'jane', 'nickname' : 'kitty'}") {'name' : 'jane', 'nickname' : 'kitty'}
We strongly advise NOT to use eval() function, and instead use literal_eval(), in case you have been asked to use eval(). This is because eval() function not only parses input string but also executes any commands within it, leaving a security vulnerability. literal_eval() only converts string to expression but does not execute it.
2. Using JSON
This is one of the most common ways to convert a string to dictionary. The string representation of dictionary can converted into a JSON string, and using JSON module, we can convert it into dictionary. We only need to replace single quotes to double quotes to do this.
import json s = "{'name' : 'jane', 'nickname' : 'kitty'}" json_acceptable_string = s.replace("'", "\"") d = json.loads(json_acceptable_string) # d = {u'name' : u'jane', u'nickname' : u'kitty'}
This method is commonly used by python-based applications and websites, to process data received from front end.
3. Using YAML
You can also use YAML module to convert such non-standard JSON strings into dictionary. In this case, you don’t need to explicitly convert the string into JSON. YAML module works on it directly.
>>> import yaml >>> s = "{'name' : 'jane', 'nickname' : 'kitty'}" >>> s "{'name' : 'jane', 'nickname' : 'kitty'}" >>> yaml.load(s) {'name' : 'jane', 'nickname' : 'kitty'}
Often websites and applications pass data from front end to back end as JSON strings, which are converted into JS objects using JSON/YAML module.
In this article, we have learnt 3 ways to convert string representation of dictionary into dictionary. They are very useful in converting any non-standard JSON to dict.
Also read:
How to Strip HTML from String Using JavaScript
How to Preload Images with jQuery
How to Make Python Dictionary from Two Lists
How to Check if JavaScript Property is Undefined
How to Get Difference Between Two Dates in JavaScript
Related posts:
How to List All Virtual Environments in Python
How to Check if Directory Exists in Python
How to Create Nested Directory in Python
How to Use Decimal Step Value for Range in Python
How to Read Inputs as Numbers in Python
How to Check if Variable is Number in Python
How to Repeat String N Times in Python
Python: Reading & Writing to Same File
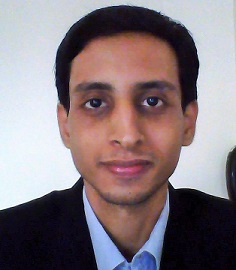
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.