JavaScript objects are popular data structures that allow you to store a lot of different types of data in a compact manner, as key-value pairs. While using JS objects, if you try to access a property that does not exist or is undefined then you will get an error and the J code execution on your page will most likely stop. So it is advisable to check if JavaScript object property is undefined before trying to access it. In this article, we will learn how to check if JavaScript object property is undefined.
How to Check if JavaScript Object Property is Undefined
There are several easy ways to check if JavaScript object property is undefined.
Let us say you have the following JS object.
test = {'a':1, 'b':2}
Let us say you want to check if property ‘c’ is assigned undefined value in JS object, then here is the command syntax on how you can do it.
if(object.property === undefined) { alert("property value is the special value `undefined`"); }
Here is how to check if property c is assigned value as undefined in test JS object defined above.
if(test.c === undefined) { alert("property value is the special value undefined"); }
If you want to check whether the property exists or not, use the following command.
if(!object.hasOwnProperty('property')) { alert("property does not exist"); }
Here is an example to check if property c exists or not in above object.
if(!test.hasOwnProperty('c')) { alert("property c does not exist"); }
If you want to check either of the above cases using the same command, that is, either the property is not declared or is set as undefined, use the following command.
if(typeof variable === 'undefined') { alert('variable is either the special value `undefined`, or it has not been declared'); }
Here is how to use the above command to check property c in our object test.
if(typeof test.c === 'undefined') { alert('test.c is either the special value `undefined`, or it has not been declared'); }
In this article, we have learnt a couple of easy ways to quickly check if an object property is defined or not, and also whether if it is assigned undefined value. Please note, these are two different cases. It is possible that an object property is defined but has been assigned undefined value.
Also read:
How to Get Difference Between Two Dates in JavaScript
How to Shuffle Array in JavaScript
How to Dynamically Create Variables in Python
How to Login to PostgreSQL Without Password
How to Store PostgreSQL Output to File
Related posts:
How to Pass Parameter to SetTimeout Callback
How to Use JavaScript Variables in jQuery Selectors
How to Remove Empty Element from JS Array
How to Get Unique Values from Array in JavaScript
How to Get ID of Element That Fired Event in jQuery
How to Generate Random String Characters in JavaScript
How to Call JS Function in IFrame from Parent Page
How to Check if String is Valid Number in JavaScript
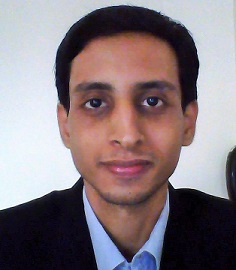
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.