Iframes provide a simple yet effective way to load one page’s contents on another page without rewriting the embedded page’s entire code on parent page. When you load a page in an iFrame, its contents as well as its functions become accessible to the parent page where it is loaded. In many cases, you may want to call a JS function defined in an IFrame’s page from the parent page.
How to Call JS Function in IFrame from Parent Page
Let us say you have a web page home.html with an IFrame with id=’myIframe’ with a function called myFunction().
<iframe id='myIframe'> ... </iframe>
You can call function myFunction() from home.html using the following command. Replace myIframe with the ID of iframe and myFunction() with the name of function you want to call.
document.getElementById('myIframe').contentWindow.myFunction();
The above command fetches the iframe using its ID. Then it uses contentWindow property of the element to call your function. Each Iframe has contentWindow property that allows you to access all function names in the iframe.
Alternatively, you can also use window.frames instead of document.getElementByID(). window.frames is an array-like object of all iframe-related information on the page.
window.frames[0].frameElement.contentWindow.myFunction();
window.frames[0] points to your iframe. Please note, if you have multiple iframes on your page, you need to replace index 0 in window.frames[0] with the appropriate index number.
You can also refer to the iframe using its name attribute as shown below. For example, if you have the following iframe with name attribute.
<iframe name='myframe'> ... </iframe>
window.frames['myframe'].frameElement.contentWindow.targetFunction();
Please note, each of the above methods will work only if you call the iframe function after it is completely loaded. If you call the function before iframe is loaded it will not work.
In such cases, it is advisable to have child iframe notify window.parent when it had finished loading and is ready to accept function calls.
In this article, we have learnt how to call JS function in iframe. They can be used to trigger functions in an iframe from its parent window.
Also read:
How to Prevent Page Refresh on Form Submit
How to Check if Object is Array in JavaScript
Regular Expression to Match URL Path
How to Split String Every Nth Character in Python
How to Reverse/Invert Dictionary Mapping in Python
Related posts:
How to Compare Arrays in JavaScript
How to Generate Random String Characters in JavaScript
How to Move Element into Another Element in jQuery
Remove Accents/Diatrics from String in JavaScript
How to Close Current Tab in Browser Window
How to Check if Variable is Array in JavaScript
How to Auto Resize IFrame Based on Content
How to Check if Variable is Undefined in JavaScript
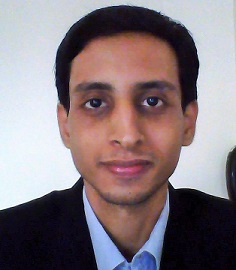
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.