Web developers rely heavily on JavaScript variables to store information and perform tasks on their websites and applications. If a variable is undefined, when you try to access it, then it may end up throwing an error and stopping your JS code execution completely. So it is always better to check if a variable is undefined before using it, at least the critical ones. There are several ways to do this. In this article, we will learn how to check if variable is undefined in JavaScript.
How to Check if Variable is Undefined in JavaScript
Before we proceed, it is important to understand a nuance about JS variable being undefined. If a JS variable is not declared and if you try to access it, its value will be undefined. If it is declared but not assigned any value or specifically assigned undefined value, even in this case it will return variable value as undefined.
Here is an example of a variable that has been declared but whose value has not been assigned. In this case when you access its type using typeof operator, it returns undefined.
var sample; //variable declared but value is undefined typeof sample; //undefined
When you check if a variable is undefined, if you are specifically looking variables that are not declared, then you need to use ‘in’ operator as shown below. All variables declared on a web page are stored in window object.
"sample" in window; // true "sample2" in window; // false
In the above example, when we use in operator with sample variable it returns true since the variable is declared but not initialized. On the other hand, when we use it with sample2 variable, it returns false since the variable is not declared.
Please note, in operator will return true if the variable is declared, irrespective of its value.
If you don’t care whether the variable is declared or not, but just whether its value is undefined or not, then you can use typeof operator. It returns undefined whether the variable is declared but unassigned or if it is specifically assigned undefined value.
if (typeof sample !== 'undefined')
Please avoid using the variable directly in if condition to check if it is undefined or not. If the variable is not defined, it will throw a ReferenceError.
if (sample2) { // ReferenceError: sample2 is not defined }
In this article, we have learnt a couple of simple ways to check if a JS variable is undefined or not. Be careful about the nuance mentioned earlier. If you need to find out whether the variable has been declared, irrespective of its value, then use in operator. If you only want to check if its value is undefined, whether it is declared or not, then use typeof operator.
So if you want to check if a variable is declared but has undefined value, you may need to use both in and typeof operators together.
In this article, we have learnt several ways to check if a JS variable is undefined or not. You can use any of them depending on your requirements.
Also read:
How to Detect Invalid Date in JavaScript
Forbidden Characters in Windows & Linux
MySQL Date Format DD/MM/YYYY in SELECT Query
How to Fix MySQL Error 1153
Create Temporary Table in MySQL SELECT Query
Related posts:
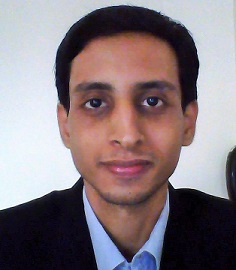
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.