JavaScript arrays are popular data structures that support many powerful features. JS also allows you to store data as objects that are key-value pairs. Many developers create array of objects to store a large volume of information in a compact and accessible manner. Sometimes you may need to remote duplicates from array of objects using JavaScript. In this article, we will learn how to remove duplicates from array of objects in JavaScript.
How to Remove Duplicates from Array of Objects JavaScript
Let us say you have the following array of objects in JavaScript. As you can see it has one duplicate item.
obj = {}; obj.arr = new Array(); obj.arr.push({place:"here",name:"stuff"}); obj.arr.push({place:"here",name:"stuff"}); obj.arr.push({place:"there",name:"morestuff"});
Let us say you want to remove duplicates from the array to create the following array.
{place:"here",name:"stuff"}, {place:"there",name:"morestuff"}
1. Using Array Filter
Here is a simple way to remove duplicate objects from our array.
const uniqueArray = obj.arr.filter((value, index) => { const _value = JSON.stringify(value); return index === obj.arr.findIndex(obj => { return JSON.stringify(obj) === _value; }); });
In the above code, we use JSON.stringify() function to convert each object into JSON string and compare the resultant JSON strings with each other to identify and remove duplicates. If two objects are identical then their JSON strings should also be equal. For this purpose, we use filter() function which creates a new array of elements that fulfill a certain condition. In our case, this condition is that there should be no duplicates of it in the array. Basically, the filter() function runs for each object one by one. For each object, it checks all duplicates in the array and returns the unique items.
There are other methods to do this also.
2. Using Temporary Array
The next method is to create a temporary array using any one of the keys, for each element. For each index, we us them to get their corresponding object from original array. At this point, our temporary array will have key-value pairs where keys are one of the keys from the objects, and value is the object itself. Then we use the values in temporary array to create new array of unique objects. This will remove duplicates since each unique key will extract only one object from the old array.
function removeDuplicates(arr_obj) { // Declare a new array let newArray = []; // Declare an empty object let uniqueObject = {}; // Loop for the array elements for (let i in arr_obj) { // Extract the title objTitle = arr_obj[i]['place']; // Use the title as the index uniqueObject[objTitle] = arr_obj[i]; } // Loop to push unique object into array for (i in uniqueObject) { newArray.push(uniqueObject[i]); } // Display the unique objects console.log(newArray); } removeDuplicates(obj);
3. Using JSON Stringify
In this case, we first convert each array item into JSON string using JSON.stringify() function. Then we create an array of these strings using map() function. Then we convert this array into a set. A set can have only unique elements so automatically, duplicate JSON strings are dropped during set creation. Finally, we get back our objects from JSON string using JSON.parse() function, and create array from set.
Here is the sample code for it.
jsonObject = obj.map(JSON.stringify); console.log(jsonObject); uniqueSet = new Set(jsonObject); uniqueArray = Array.from(uniqueSet).map(JSON.parse); console.log(uniqueArray);
In this article, we have learnt how to remove duplicates from array of objects in JavaScript.
Also read:
How to Listen to Variable Changes in JS
How to Reset Primary Key Sequence in PostgreSQL
How to Pass Parameter to SetTimeout Callback
How to Generate Random String in JS
How to Fix PostgreSQL Error Fatal Role Does Not Exist
Related posts:
How to Get Position of Element in HTML
How to Detect When User Leaves Page
How to Display Local Storage Data in JavaScript
How to Find Sum of Array of Numbers in JavaScript
How to Convert Array to Object in JavaScript
How to Get Unique Values from Array in JavaScript
How to Detect Invalid Date in JavaScript
How to Get Client IP Address Using JavaScript
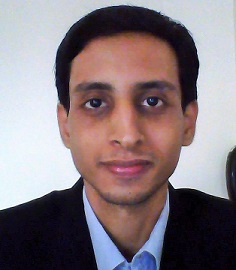
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.