Local Storage provides a great way to store data locally on your website visitor’s browsers. This data is retained even when the page is refreshed and does not get reset. Often, while working with local storage data, you will need to be able to retrieve and display local storage data, using JavaScript. In this article, we will learn how to do this.
How to Display Local Storage Data in JavaScript
Here are the steps to display local storage data in JavaScript.
Local Storage in web browsers can be accessed using LocalStorage object. It offers two methods setItem() and getItem() to save and retrieve data respectively.
Here is the syntax to store data in local storage.
localStorage.setItem(variable_name,variable_value)
Here is a simple example to store string ‘abc’ in Local storage variable ‘def’.
var a='abc'; localStorage.setItem('def',a);
You can use setItem() function to store not just strings but also numbers, arrays, objects in local storage.
Here is an example to store array and object as local storage data.
var b=[1,2,3,4]; var c={'b':123,'f':3434}; localStorage.setItem('array',b); localStorage.setItem('obj',c);
Now if you want to display local storage item, you can do it in two ways – either you display value of a single specific variable, or you can display values of all variables. We will look at both these methods.
If you want to display local storage data stored in a specific variable, use the following syntax for getItem() function.
localStorage.getItem(variable_name)
Here is an example to get value of variables stored earlier.
var temp=localStorage.getItem('a'); alert(temp); //output 'abc' var temp=localStorage.getItem('b'); var temp=localStorage.getItem('c');
If you want to display values of all local storage variables, just run a loop through localStorage object. It provides a couple of inbuilt properties to help you do this. localStorage.length contains count of variables, and localStorage.key() returns the variable name.
for (var i = 0; i < localStorage.length; i++){ alert( localStorage.getItem(localStorage.key(i))); }
In the above code, we loop through the localStorage variables one by one and display their values using alert boxes.
Also read:
How to Enable, Disable & Install Yum Plugins
How to Delete Root Emails in Linux
How to Check if Element is Visible in Viewport
How to Check if Element is Hidden in JavaScript
How to Stay on Same Tab After Page Refresh
Related posts:
How to Get Duplicate Values in JS Array
How to Get Array Intersection in JavaScript
How to Remove Empty Element from JS Array
How to Check if Element is Hidden in JavaScript
How to Get Random Number Between Two Numbers in JavaScript
How to Capitalize First Letter in JavaScript
How to Call JS Function in IFrame from Parent Page
How to Disable Clicks in IFrame Using JavaScript
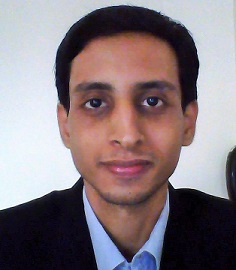
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.