These days many websites use long pages to display a lot of content in one place. It can be tedious to manually scroll to bottom of such long pages and you may want to provide a scroll to bottom feature or automatically scroll to bottom of page. This can be easily done using plain JavaScript and does not require any third party library. In this article, we will learn how to automatically scroll to bottom of page in JS.
How to Automatically Scroll to Bottom of Page in JS
You can automatically scroll to bottom of page by adding the following JavaScript code.
window.scrollTo(0, document.body.scrollHeight);
It uses scrollTo() function to scroll down the document, to a distance equal to height of document.body.
If you have nested DOM elements on your page, the document object may not scroll. Let us say you have a scrollable div on your page.
<div id='scrolling_div'>...</div>
In such cases, you need to call the scrollTo() function on the scrollable div element, as shown below.
window.scrollTo(0, document.querySelector("#scrolling_div").scrollHeight);
The above codes will automatically scroll to bottom of page, whenever it is loaded. If you want to call the function at the click of a button, you can add it to the onClick event handler of a clickable element like button or anchor tag.
<button id='scroll_button' onClick='scroll_to_bottom()'>Click me</button>
Thereafter you can add the following JS code.
function scroll_to_bottom(){ window.scrollTo(0, document.body.scrollHeight); }
If none of the above methods work for you, then try using the following code.
element.scrollTop = element.scrollHeight
Applying the above code to our scrollable div
document.querySelector("#scrolling_div").scrollTop = document.querySelector("#scrolling_div").scrollHeight
In this article, we have learnt how to automatically scroll to bottom in JavaScript. You can use it to make your web pages easily scrollable and help users navigate through your content.
Also read:
How to Change Image Source in jQuery
How to Check for Hash in URL in JavaScript
How to Check if File Exists in NodeJS
How to Load Local JSON File
How to Show Loading Spinner in jQuery
Related posts:
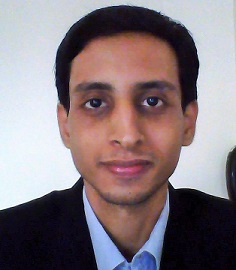
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.