Often web developers use arrays in JavaScript to store data. Sometimes you may need to duplicate arrays. It can be tedious to create an empty array, and iterate through original array to copy each element into the new array. There are several easy and fast ways to clone arrays in JavaScript. In this article, we will learn several simple ways to duplicate arrays in JavaScript.
How to Clone Array in JavaScript
Let us say you have the following large array.
arr = [1,2,3,4...,1000]
Here are some of the most common and fast ways to clone arrays in JavaScript.
1. Using slice
Slice() function is typically used to extract part of array or string but it can also be used to duplicate an array quickly. Here is the command to clone array arr.
arr2 = arr.slice();
In many cases, using slice(0) can be faster than using slice().
arr2 = arr.slice(0);
Please note, this will only create a shallow copy, meaning if your array consists of references to objects, then the duplicate array will contain references to the same objects. It won’t create new objects to be referenced by duplicate array.
2. Using While Loop
Although looping may seem tedious, in some browsers they are optimized and can give fast results. Here is an example to duplicate an array using while loop.
i = arr.length; while(i--) arr1[i] = arr[i];
3. Using spread operator
You can also use spread operator (…) to clone an array real quick, as shown below. In fact, it is the fastest way to clone array in most cases.
arr1 = [...arr];
Spread operator allows you to quickly copy a part or all of an existing array.
4. Using Concat
Although concat() function is typically used to concatenate strings and arrays, you can also use it to duplicate arrays. Here is the command to clone an array in JS.
var arr1 = [].concat(arr);
In this article, we have learnt several simple and quick ways to clone arrays in JS. Among them, using spread operator and slice() function are the fastest ways to clone arrays. They are especially useful if you want to copy large arrays. Nevertheless, you can use any of the above methods depending on your requirement.
Also read:
How to Check MySQL Storage Engine Type
How to Use LIKE Operator for Multiple Values
How to Increase Import File Size Limit in PHPMyAdmin
How to Add Column After Another Column in MySQL
How to Retrieve MySQL Username and Password
Related posts:
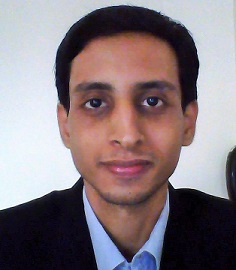
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.