Often web developers need to determine if a user is accessing their websites and applications using laptop or touchscreen device like mobiles and tablets. You can easily do this using plain JavaScript, although there are several third-party libraries to help you do this. In this article, we will learn how to detect touchscreen device in JavaScript.
How to Detect Touchscreen Device in JavaScript
Browsers on desktops and laptops do not have touch capabilities while those on mobile devices do. So we will use JavaScript to check if the browser has touch capabilities. If so, then the user is accessing your website using touchscreen device
1. Using JavaScript
Every web browser has inbuilt object called navigator that stores a lot of information about browser as well as the user. It has MaxTouchPoints that returns the maximum number of touch points supported on the browser. For touchscreen devices this value is greater than 0. We also check if ontouchstart property is supported by the browser. So you can use the following function to check if touch is supported in web browser. It will return true if touch is supported on web browser, else return false.
function is_touch_device() { return (('ontouchstart' in window) || (navigator.maxTouchPoints > 0) || navigator.msMaxTouchPoints > 0)); }
2. Using Modernizr
Modernizr is a light-weight JS library that allows you to check if browser supports different kinds of features, including touch. It basically adds different types of HTML classes to each element, depending on the presence or absence of features in the user’s web browser. You can use CSS or JS to work these classes. Here is an example to hide an HTML element that does not support touch.
$('html.touch #popup').hide();
In this article, we have learnt a couple of simple ways to detect touchscreen device using JavaScript.
Also read:
How to Fix ‘Server Quit Without Updating PID’ MySQL Error
How to Change Apache Config Without Restarting
How to Change NGINX Config Without Restarting
How to Get Selected Text from Dropdown Using jQuery
How to Get Data-ID Attribute in jQuery
Related posts:
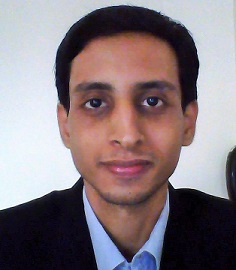
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.