Web developers use data attributes to store different types of information in HTML DOM elements. Often you may need to get data attributes from your HTML elements. In this article, we will learn how to get data-id attribute in jQuery/JavaScript.
How to Get data-id attribute in jQuery / JavaScript
Let us say you have the following HTML element.
<a id='myAnchor' data-id='myLink' data-text='hello world'>Hello World</a>
There several ways to get data-id attribute in jQuery.
1. Using attr()
You can easily get data-id attribute using attr() function. You need to use the right selector to select the element, and then call attr() function for it. The following command will return the text ‘myLink’.
$('#myAnchor').attr("data-id");
You can also use the same method to get other data attributes also. Here is a command to get data-text attribute.
$('#myAnchor').attr("data-text");
Please note, in attr() function, you need to use the entire data attribute’s name, including ‘data-‘ part. Mentioning ‘id’ alone will not work.
2. Using prop()
prop() function is similar to attr() and has the same syntax. You can get data attributes using prop() function as shown below.
$('#myAnchor').prop("data-id"); //returns myLink $('#myAnchor').prop("data-text"); //returns hello world
Please note, in prop() function also, you need to use the entire data attribute’s name, including ‘data-‘ part. Mentioning ‘id’ alone will not work.
3. Using data() function
You can also use data() function to get a specific data attribute’s value as shown below. It is supported since jQuery>=1.4.3
$('#myAnchor').data("id"); //returns myLink $('#myAnchor').data("text"); // returns hello world
Please note, while using data() function, you should not mention ‘data-‘ inside data() function. Otherwise it will not work. You need to mention the part after ‘data-‘.
4. Using JavaScript
You can also use plain JavaScript to get data-id attribute as shown below. First, we select the element using getElementById() function and then get data attribute using getAttribute() function.
var link = document.getElementById('myAnchor'); var data_id = link.getAttribute('data-id'); /
In this article, we have learnt several ways to get data-id attribute using jQuery as well as using JavaScript. Please keep in mind the difference between using attr()/prop() and data()
Also read:
How to Check if Image is Loaded in jQuery/JavaScript
How to Fix Ambiguous Column Names in MySQL
How to List All Foreign Keys to Table in MySQL
How to Check if Row Exists in MySQL
How to Center Div Using jQuery
Related posts:
How to Detect Mobile Device Using jQuery
How to Call JS Function in IFrame from Parent Page
How to Generate Random Color in JavaScript
How to Pass Parameter to SetTimeout Callback
How to Convert Comma Separated String into JS Array
How to Persist Variables Between Page Load
How to Measure Time Taken by JS Function to Execute
How to Count Frequency of Array Items in JavaScript
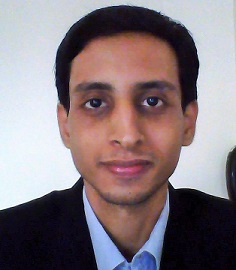
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.