These days almost every website uses images, and if they are not loaded or loaded improperly, then it spoils the user experience. So some web developers choose to check if the images on their web pages are loaded or not. This can be done using JavaScript and third-party JavaScript libraries like jQuery. In this article, we will learn how to check if image is loaded in jQuery.
How to Check if Image is Loaded in jQuery / JavaScript
Let us say you have the following image tag on your webpage.
<img id='myImage' src='/images/test.png'/>
1. Using jQuery
You can easily check if this image is loaded using jQuery.
$("<img/>") .on('load', function() { console.log("image loaded correctly"); }) .on('error', function() { console.log("error loading image"); }) .attr("src", $(originalImage).attr("src")) ;
In the above code, we attach event listeners to load and error events for our image tag. If the image is loaded properly, load event is triggered, else it triggers error event. Accordingly, it will display a message in console.
Please note, sometimes load event may not be triggered if the image is loaded from the cache. In such cases, it is better to invalidate the cache from server or using JS so that the next time a user loads your page, these events are triggered properly. This is a common problem in browsers like Chrome which heavily rely on cache contents to load websites.
2. Using JavaScript
You can also use plain JavaScript for this purpose. Here is a simple function to check if image is loaded or not.
function check_image(img) { if (!img.complete) { return false; } if (img.naturalWidth === 0) { return false; } return true; }
In the above function, when the image is loaded its onload event is called and most browsers should be able to check the ‘complete’ property value of image to find out if it is properly loaded or not.
However, if a browser is unable to process the above property, then it can read naturalWidth/naturalHeight property of the image. When an image is not loaded properly, either of these values will be zero.
You can use the above function to loop through all images on your web page and check if each of them is loaded properly or not.
AddEvent(window, "load", function() { for (var i = 0; i < document.images.length; i++) { if (!check_image(document.images[i])) { document.images[i].style.visibility = "hidden"; } } });
We run the above code when the page is loaded. It loops through all the images on your page, which are stored in document.images object by default. Based on the value of the check_image() function, we hide/display images so that broken images are automatically hidden.
In this article, we have learnt how to check if image is loaded correctly or not. You can use it to detect broken images on your websites quickly.
Also read:
How to Fix Ambiguous Column Names in MySQL
How to List All Foreign Keys to Table in MySQL
How to Check if Row Exists in MySQL
How to Center Div Using jQuery
How to Select Element Using Data Attribute in jQuery
Related posts:
Remove Unicode Characters from String
How to Persist Variables Between Page Load
How to Detect if Device is iOS
How to Get Client IP Address Using JavaScript
How to Check for Hash (#) in URL Using JavaScript
How to Add 1 Day to Current Date
How to Get URL Parameters Using jQuery or JavaScript
How to Detect Internet Connection is Offline in JavaScript
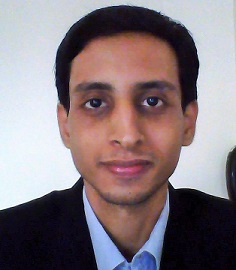
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.