Sometimes when users are browsing your website or using your web application, their internet connection might get disconnected and they may be unable to use it. In such cases, they may think something is wrong with your website or application and leave. So it is advisable to check internet connection from your website or app itself and inform users if they get disconnected. This improves your website/app’s user experience and retains visitors longer. In this article, we will learn how to detect internet connection is offline in JavaScript.
How to Detect Internet Connection is Offline in JavaScript
Almost all major web browsers support window.navigator.onLine property, along with online and offline window events. If a system is offline, window.navigator.onLine will return false, else it will return true.
Use the following snippet to check if internet connection is offline in user’s web browser.
console.log('You are ' + (window.navigator.onLine ? 'on' : 'off') + 'line');
You can run the above code when your web page loads so that it is one of the first things to be checked before other tasks are processed.
window.addEventListener("load", (event) => { console.log('You are ' + (window.navigator.onLine ? 'on' : 'off') + 'line'); });
Alternatively, you can call it at regular intervals using setInterval() function to make sure that your users are always online. The following code runs every 5 minutes. You can customize it as you need it.
setInterval(function () {console.log('You are ' + (window.navigator.onLine ? 'on' : 'off') + 'line');}, 300000);
You can also call the internet connection check asynchronously so that it does not affect rest of the JS code on your web page.
window.addEventListener("load", async (event) => { console.log('You are ' + (window.navigator.onLine ? 'on' : 'off') + 'line'); });
window.navigator.Online also supports a couple of events, for online and offline conditions. Use the following code snippet to trigger event handlers when user becomes online or offline.
window.addEventListener('online', () => console.log('You Became online')); window.addEventListener('offline', () => console.log('You Became offline'));
If you want to trigger the checking of internet connection at the click of a button, add the following event handler.
document.getElementById('statusCheck').addEventListener('click', () => alert('window.navigator.onLine is ' + window.navigator.onLine));
Attach the above event handler to the following button.
<button id="statusCheck">Check internet connection</button>
Now when you click the above button, it will use the event handler to check internet connection.
But please note, if window.navigator.onLine is true, it need not necessarily mean that your system is connected to internet. Even if it is connected to a virtualization software with virtual ethernet adapters, it will still return true. In such cases, you may need to use additional means to check if a system is online or not.
On the other hand, if window.navigator.onLine is false, it means your system is definitely offline. So you should check for this value to confirm that internet connection is online/offline.
In this article, we have learnt how to detect internet connection is offline. There is no third-party library or API required for this. You can add this code to your website or app to check if your users are offline or not.
Also read:
How to Remove All Child Elements of DOM Node in JS
How to Check if Variable Exists or is Defined in JS
How to Convert UTC to Local Time in JS
How to Use Variable Number of Arguments in JS Function
How to Add 1 Day to Current Date
Related posts:
How to Convert Comma Separated String into JS Array
How to Use Variable As Key in JavaScript Object
How to Get Nested Object Keys in JavaScript
How to Display Local Storage Data in JavaScript
How to Convert UTC Date Time to Local Date Time in JS
How to Convert Array to Object in JavaScript
How to Check if Object is Array in JavaScript
How to Return Response from Asynchronous Call in JavaScript
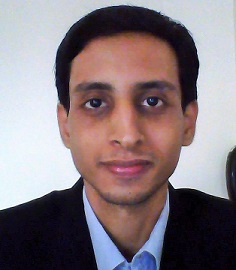
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.