These days many website URLs contain Hash (#) to point browser to specific part of a web page or website. Sometimes you may need to check for hash in URL. For example, you may want to run a code snippet only if the current page URL has hash in it. You can easily do this using plain JavaScript, without using any third-party library. In this article, we will learn how to check for Hash in URL using JavaScript.
How to Check for Hash (#) in URL Using JavaScript
Most modern browsers already provide window interface that allows you to easily determine whether hash exists or not. window.location returns the current web URL and window.location.hash returns true if the current URL contains hash, else it returns false.
if(window.location.hash) { // Fragment exists } else { // Fragment doesn't exist }
If your URL is stored as a string variable, say url, you can use the index() function to check for presence of hash sign in it.
if (url.indexOf('#') !== -1) { // url contains a # }else{ // url does not contain a # }
If you want to obtain the URL fragment after hash, you can do it using the split() function as shown below.
hash = url.split('#')[1];
In this article, we have learnt how to check for hash in URL in JavaScript.
Also read:
How to Check if File Exists in NodeJS
How to Load Local JSON File in JS
How to Show Loading Spinner in jQuery
How to Convert String to Boolean in JavaScript
How to Convert Decimal to Hex in JS
Related posts:
How to Break ForEach Loop in JavaScript
How to Detect Internet Connection is Offline in JavaScript
How to Compare Two Dates Using JavaScript
How to Check if Element is Hidden in JavaScript
How to Close Current Tab in Browser Window
How to Check if Object is Empty in JavaScript
How to Allow only Alphabet Input in HTML Text Input
How to Format Number as Currency String
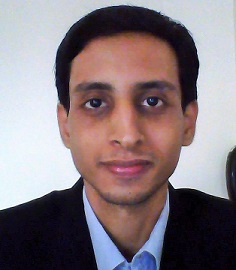
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.