Sometimes web developers may need to change image source on their web pages. It may be because of website redesign or they may want to rotate images in a placeholder DOM element. These cases can be easily handled using jQuery, instead of changing HTML code. In this article, we will learn how to change image source in jQuery.
How to Change Image Source in jQuery
Let us say you have the following image tag on your web page.
<img id="my_image" src="/images/test.jpg" alt="alt text here" />
Let us say you want to change the image source to /images/new/test2.jpg, then we will need to change the src attribute of above img tag. You can do this using attr() function that you can call on any DOM element by specifying its selector, and mention the attribute to be changed along with its new value.
$("#my_image").attr("src","/images/new/test2.jpg");
Please note, you need to specify the complete new path (relative or absolute) of image, even if it is in the same folder. Attr() function will completely overwrite the specified attribute with new value.
Change Image Source on Click
If you want to change the image source on click of a button, you can specify the above code in a click event handler as shown below.
$('#my_image').on({ 'click': function(){ $('#my_image').attr('src','/images/new/test2.jpg'); } });
If you want to rotate the images, you can modify the above function to change image source, depending on the current source.
$('img').on({ 'click': function() { var src = ($(this).attr('src') === '/images/img1.jpg') ? '/images/img2.jpg' : '/images/img1.jpg'; $(this).attr('src', src); } });
In the above code, when user clicks, the function checks the image’s current source. If it is pointing to img1.jpg, it is replaced by path to img2.jpg, else it is replaced by path to img1.jpg.
In this article, we have learnt how to change image source in jQuery.
Also read:
How to Check for Hash in URL Using JavaScript
How to Check if File Exists in NodeJS
How to Load Local JSON File
How to Show Loading Spinner in jQuery
How to Convert String to Boolean in JS
Related posts:
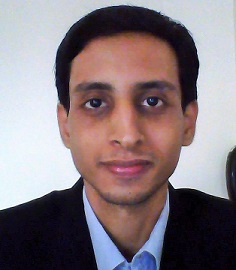
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.