Often developers use anchor links to help users navigate to different sections of the page. But when a user clicks one of these links the browser directly jumps to the corresponding section. This can be a suboptimal user experience. In this article, we will learn how to smooth scroll on clicking links. You can do this using JavaScript or jQuery.
How to Smooth Scroll on Clicking Links
There are several ways to smooth scroll on clicking links.
1. Using JavaScript
Most modern browsers like Chrome, Firefox, Edge & Safari offer native ways to do smooth scrolling. You can easily add smooth scrolling by adding following JS function.
document.querySelectorAll('a[href^="#"]').forEach(anchor => { anchor.addEventListener('click', function (e) { e.preventDefault(); document.querySelector(this.getAttribute('href')).scrollIntoView({ behavior: 'smooth' }); }); });
It adds a click event listener to each link which href begins with ‘#’ which indicates a jump link not a URL link. When a jump link is clicked, the function will retrieve the value of href attribute and scroll it into view smoothly.
2. Using jQuery
You can also use jQuery to do the same thing. Here we attach a click event listener to document object. Every time there is a click on the page, it will check if the click was made to jump link. If so, then it calls animate() function to scroll to the href attribute’s value, in smooth manner.
$(document).on('click', 'a[href^="#"]', function (event) { event.preventDefault(); $('html, body').animate({ scrollTop: $($.attr(this, 'href')).offset().top }, 500); });
In this article, we have learnt how to smooth scroll on clicking links.
Also read:
How to Import SQL File in MySQL Using Command Line
How to Change Date Format in jQuery UI Date Picker
How to Fix NGINX Bind to 0.0.0.0:80 Failed Error
How to Fix NGINX Unable to Load SSL Certificate
How to Detect Touchscreen Device in JavaScript
Related posts:
How to Escape HTML Strings With jQuery
How to List Properties of JS Object
How to Deep Clone Objects in JavaScript
How to Add Event to Dynamic Elements in JavaScript
How to Render HTML in TextArea
Return False vs PreventDefault in JavaScript
How to Clear HTML5 Canvas for Redrawing in JavaScript
How to Select Element by Data Attribute in jQuery
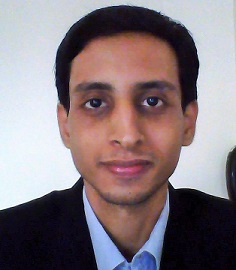
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.