JavaScript objects allow you to store diverse types of data in a compact manner as key-value pairs. They can be easily converted into JSON and vice versa, making them very versatile and preferred format for web developers. Often while using JS objects you may need to view its properties. In this article, we will learn how to list properties of JS object.
How to List Properties of JS Object
Most modern browsers provide Object.keys() method that returns all the properties.
Let us say you have the following JS object.
var test_obj = {1:'a',2:'b',3:'c'};
Here is how you can get list of properties of this object.
var keys = Object.keys(test_obj);
If you want to directly call this keys() method on any object, you can define a prototype for it.
var Object.prototype.keys = function(obj){ var keys = []; for(var key in obj){ keys.push(key); } return keys; } OR var Object.prototype.keys = function(obj){ return Object.keys(obj); }
Then you can directly call test_obj.keys to get list of properties of this object.
You can also loop through the properties one by one, in case you are looking for any specific property or want more control over the output.
for (var key in obj) { if (obj.hasOwnProperty(key)) { /* some code here */ } }
The above code will iterate over the object’s attribute names. We have used hasOwnProperty() function to iterate only over Object’s own properties, instead of all attributes included in Object’s prototype chain.
In this article, we have learnt how to display properties of JS object.
Also read:
How to Detect if Device is iOS
How to Count Character Occurrence in JS String
How to Add Auto Increment ID to MySQL Table
JS File Upload Validation
How to Print Contents of Div in JavaScript
Related posts:
How to Disable Clicks in IFrame Using JavaScript
How to Get Array Intersection in JavaScript
How to Get Position of Element in HTML
How to Parse URL into Hostname & Pathname in JS
How to Detect Touchscreen Device in JavaScript
How to Get URL Parameters Using jQuery or JavaScript
How to Convert Comma Separated String into JS Array
How to Display Local Storage Data in JavaScript
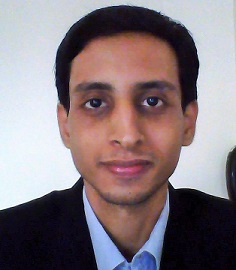
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.