Sometimes web developers need to split URL into hostname and pathname in JavaScript. In this article, we will learn how to do this easily. We will see a couple of ways to parse URL into hostname & pathname in JS.
How to Parse URL into Hostname & Pathname in JS
There are two ways to parse URL – either it is the URL of current web page’s address or it is a plain string.
1. Parse Current URL
If you want to parse your web page’s current URL into hostname and pathname you can easily do this using window.location or just location object. Both of them have hostname and pathname properties that automatically store the hostname and pathname of current URL. Let us say your current URL is http://example.com/product/abc. Here is the command to retrieve hostname of current URL in web browser.
host = window.location.hostname; //returns www.example.com OR host = location.hostname; //returns www.example.com
Similarly, here is the command to retrieve pathname of current URL.
path = window.location.pathname; //returns /product/abc OR path = location.pathname; //returns /product/abc
2. Parse URL String
If your URL is a plain string, then you can use the URL() constructor to convert it into URL. This returns an object with two properties hostname and pathname that contain hostname and pathname of URL respectively.
var url = new URL("http://www.example.com/product/abc"); console.log(url.hostname); //returns www.example.com console.log(url.pathname); //returns /product/abc
In this article, we have learnt how to parse URL into hostname and pathname in JavaScript.
Also read:
How to Remove Element By ID in JavaScript
How to Write to File in NodeJS
How to Export JS Array to CSV File
How to Convert Unix Timestamp to Datetime in Python
How to Smooth Scroll on Clicking Links
Related posts:
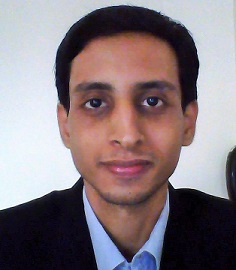
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.