JavaScript allows you to work with images and DOM elements in web pages. You can do many useful image transformations and manipulations using JavaScript. Often you may need to get image size & width using JavaScript. In this article, we will learn how to do this. It is useful for getting dimensions of images on web pages.
How to Get Image Size & Width Using JavaScript
Let us say you have the following image tag on your web page.
<html> <body> <img id="myImage" src="http://www.google.com/intl/en_ALL/images/logo.gif"/> </body> </html>
There are several ways to get image dimensions on your page. Here is a simple code to get image dimensions using plain JavaScript.
var img = document.getElementById('myImage'); var width = img.clientWidth; var height = img.clientHeight;
In the above code, we first get a handle for the image, using its ID. Then we use clientWidth and clientHeight attributes to get the width and height of images. clientWidth and clientHeight are DOM properties that show the dimensions of DOM element, excluding margins and borders.
You can also programmatically check the dimension of image by loading it dynamically and using Image() API function for this purpose. Here is a sample code to do it.
img = new Image(); img.onload = function() { alert(this.width + 'x' + this.height); } img.src = 'http://www.google.com/intl/en_ALL/images/logo.gif';
In this case, we use the width and height properties of images to get dimensions of image. If you want to get the original width and height of image used in the tag, you need to use naturalWidth and naturalHeight instead, as shown below. clientWidth and clientHeight show the size of the image as displayed in your browser.
var img = document.getElementById('myImage'); var width = img.naturalWidth; var height = img.naturalHeight;
If you are using jQuery, you need to call the following code after your page is loaded.
$(document).ready(function() { $("#myImage").load(function() { alert($(this).height()); alert($(this).width()); }); });
In the above code, we have selected the image using its ID attribute. Thereafter, we have called width() and height() functions to get its dimensions.
If you need to get dimensions of multiple images, you will need to loop through them and get the dimension of each image, one at a time. Here is a simple way to get the dimensions of all images on your web page.
$(document).ready(function() { $("img").each(function() { $(this).load(function() { alert($(this).height()); alert($(this).width()); }); }); });
In this article, we have learnt a couple of simple ways to get the width and height of image using JavaScript.
Also read:
How to Use Multiple jQuery Versions on Same Page
How to Convert Form Data to JS Object using jQuery
How to Detect Mobile Device Using jQuery
How to Bind Event to Dynamic Element in jQuery
How to Find Sum of Array of Numbers in JavaScript
Related posts:
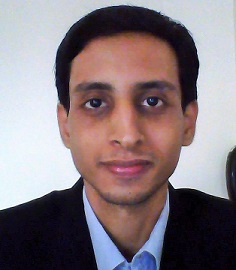
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.