Some people access websites via desktop web browsers while others do it using mobile phones. It is important to determine if a visitor is using your website or app via mobile or desktop device. It allows you to personalize user experience easily. In this article, we will learn how to detect mobile device using jQuery.
How to Detect Mobile Device Using jQuery
One of the simplest ways to detect mobile device is by using plain JavaScript (not jQuery) as shown below. When a user visits your website or web application, their browser information is stored in navigator.userAgent variable. Depending on the mobile platform, it contains a different string. We can use the test() function to check if it contains any of the substrings that can be used to identify the web browser.
if( /Android|webOS|iPhone|iPad|iPod|BlackBerry|IEMobile|Opera Mini/i.test(navigator.userAgent) ) { // some code pertaining to mobile devices }
In the above code, we check if the userAgent variable contains any of the strings such as Android, webOS, iPhone, etc. that can be used to identify the mobile device.
The above test function returns true if the userAgent variable contains any of the specified substrings, else it returns false.
On some Firefox browsers, the above method may not work.
So here is another way to do the same thing. It involves using CSS and jQuery.
First, we add the following CSS media query to our web page. Replace some-element with an element from your web page that can be hidden without any problem.
/* Smartphones ----------- */ @media only screen and (max-width: 760px) { #some-element { display: none; } }
Add the following jQuery code to your HTML page.
$( document ).ready(function() { var is_mobile = false; if( $('#some-element').css('display')=='none') { is_mobile = true; } // now I can use is_mobile to run javascript conditionally if (is_mobile == true) { //Conditional script here } });
In the above code, we basically use CSS media query to hide an element on your page if it is loaded in mobile device. Our jQuery code checks if this element is visible or not. If it is not visible, it means the page is loaded on mobile device and this information is used to set is_mobile variable to true. Else it is set to false.
These days modern web browsers also provide a JavaScript API (window.matchMedia) to directly detect if the web page is loaded in mobile device, using the following code. In the following code, isMobile variable is set to true if the web page is loaded in mobile device, else it is set to false.
$(function() { let isMobile = window.matchMedia("only screen and (max-width: 760px)").matches; if (isMobile) { //Conditional script here } });
In this article, we have learnt how to detect mobile device using jQuery. You can use this to customize your website and applications, depending on whether they are loaded in mobile or desktop browsers.
Also read:
How to Bind Event to Dynamic Element in jQuery
How to Find Sum of Array Numbers in JavaScript
Remove Unicode Characters from String
Remove Accents/Diatrics from String in Python
Remove Accents/Diatrics from String in JavaScript
Related posts:
How to Check if Variable is Array in JavaScript
How to Change Element's Class in JavaScript
How to Detect When User Leaves Page
How to Remove All Child Elements of DOM Node in JS
How to Remove Duplicates from Array of Objects JavaScript
How to Find Sum of Array of Numbers in JavaScript
How to Deep Clone Objects in JavaScript
How to Get Client IP Address Using JavaScript
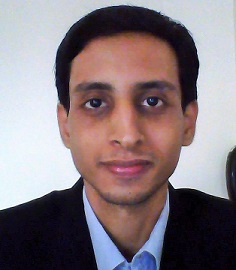
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.