Web development technologies such as HTML, CSS & JS allow you to specifically position DOM elements as per your requirements. In some cases, you may need to get position of element in HTML. There are several ways to do this. In this article, we will learn how to get position of element in HTML.
How to Get Position of Element in HTML
Let us say you have the following element.
<div id='myDiv'> ... </div>
One of the simplest JavaScript-based, cross-browser method to get the position of this div is using getBoundingClientRect() function. First we need to get hold of the specific element whose position we want to determine.
var element = document.getElementById("myDiv");
Then you need to call getBoundingClientRect() function on the element, as shown below.
var rect = element.getBoundingClientRect();
The above function stores the top, left, bottom and right positions in an JS object and assigns it to variable rect.
Then you can easily retrieve these values using these properties as shown below.
console.log(rect.top, rect.right, rect.bottom, rect.left);
Some browser also return height and width properties.
Similarly, you can also obtain the position of other HTML elements such as body.
var bodyRect = document.body.getBoundingClientRect();
Please note, the position values returned are relative to the viewport. If you need them relative to another element, such as HTML body, you need to subtract the position of one element from the other to calculate the offset values.
var bodyRect = document.body.getBoundingClientRect(), elemRect = element.getBoundingClientRect(), offset = elemRect.top - bodyRect.top; alert('Element is ' + offset + ' vertical pixels from <body>');
You can also use third party libraries like jQuery to get position of an element. In fact, third-party libraries provide plenty of out-of-the-box functions to quickly calculate element position relative to viewport, another element, parent element, etc.
Here is a jQuery function position() that returns top & left position of element, relative to parent element
var x = $('#myDiv').position(); alert("Top: " + x.top + " Left: " + x.left);
You can get the absolute top & left positions of your element using offset function.
var position = $('#myDiv').offset(); // e.g. position = { left: 40, top: 500 }
In this article, we have learnt how to get the position of HTML elements using JavaScript as well as jQuery.
Also read:
How to Get Duplicate Values in JS Array
How to Create Multiline Strings in JavaScript
How to Split String By Particular Character
How to Read Local Text File in Web Browser
How to Encode String to Base64 in JavaScript
Related posts:
How to Get Array Intersection in JavaScript
How to Get Property Values of JS Objects
How to Get Image Size & Width Using JavaScript
How to Scroll to Bottom of Div
How to Get ID of Element That Fired Event in jQuery
How to Use Variable in Regex in JavaScript
How to Remove Empty Element from JS Array
How to Check if Element is Hidden in JavaScript
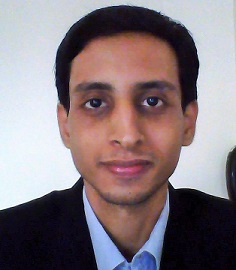
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.