JavaScript allows you to do many fun things with strings and text data. Sometimes you may want to create a multiline string in HTML/JavaScript. By default, if you type multiline string within single or double quotes it will give an error since JavaScript expects each string to end on the same line, by default. In this article, we will learn how to create multiline strings in JavaScript.
How to Create Multiline Strings in JavaScript
There are many ways to create multiline strings in JavaScript. We will look at a couple of simple ones.
1. Using backticks
You can create multiline strings in JavaScript by enclosing your entire string in backticks, instead of using double quotes or single quote. Here are a couple of examples.
var htmlString = `Say hello to multi-line strings!`; var html = ` <div> <span>Some HTML here</span> </div> `;
The above feature is a result of template literals introduced in ECMAScript (ES6). It also supports variable interpolation in strings.
When you print such strings in console, or HTML they will be displayed as a multiline string.
console.log(htmlString); Say hello to multi-line strings! console.log(html); <div> <span>Some HTML here</span> </div>
2. Using backslash
You can also use backslash to create multiline strings, as shown below. In this case, you can use double and single quotes, instead of using backticks.
var htmlString = "Say hello to \ multi-line \ strings!"; var html = " \ <div> \ <span>Some HTML here</span> \ </div> \ ";
You can also store strings as array elements and join them using backslash to get a multiline string, but the above methods are faster, especially for long strings.
In this article, we have learnt a couple of simple ways to store multiline strings in JavaScript. You can use them as per your requirement, as a part of a bigger function or module.
Also read:
How to Split String by Character in JavaScript
How to Read Local Text File in Browser Using JavaScript
How to Encode String to Base64 in JavaScript
How to Get Length of JavaScript Object
How to Get Client IP Address Using JavaScript
Related posts:
How to Set/Unset Cookie With jQuery
How to Pass Parameter to SetTimeout Callback
How to Get Property Values of JS Objects
How to Use JavaScript Variables in jQuery Selectors
How to Calculate Age from DOB in JavaScript
How to Count Frequency of Array Items in JavaScript
How to Detect When User Leaves Page
How to Add Days to Date in JavaScript
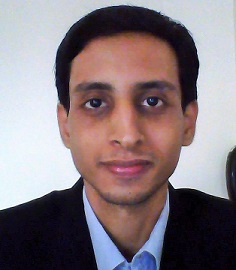
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.