JavaScript arrays are powerful data structures used by many web developers. They offer tons of functions and features. Often you may need to count frequency of array items in JavaScript. In this article, we will learn how to do this easily.
How to Count Frequency of Array Items in JavaScript
Let us say you have the following array.
var arr = [5, 5, 3, 2, 2, 2, 2, 2, 9, 4];
1. Using JavaScript
We will use a JavaScript object to store the counts of each element. We will store the array item as key and its count as the property.
var counts = {};
We will loop through the array items one by one and do one of the following:
- If the item is not present as a key, we will add it to JS object counts.
- If the item is already present in the JS object, we increment its count.
for (const num of arr) { counts[num] = counts[num] ? counts[num] + 1 : 1; } console.log(counts); //{'5':2,'3':1,'2':5,'9':1,'4':1}
2. Using Underscore or Lodash
If you are using third-party libraries like Underscore or Lodash, you can use count() function which is available directly out of the box.
_.countBy(array);
Here is an example.
_.countBy([5, 5, 3, 2, 2, 2, 2, 2, 9, 4]) => Object {2: 5, 3: 1, 4: 1, 5: 3, 9: 1}
In this article, we have learnt how to get frequency count of array items using JavaScript.
Also read:
How to Allow Only Alphabet Input in HTML Textbox
How to Allow Only Numeric Input in HTML Textbox
How to Disable Scrolling in HTML/JavaScript
How to Change Sudo Password Timeout in Linux
How to Reset WordPress Admin Password via MySQL
Related posts:
How to Allow only Alphabet Input in HTML Text Input
How to Use JavaScript Variables in jQuery Selectors
How to Use Variable in Regex in JavaScript
How to Persist Variables Between Page Load
How to Get Unique Values from Array in JavaScript
How to Get Length of JavaScript Object
How to Change Element's Class in JavaScript
How to Remove All Child Elements of DOM Node in JS
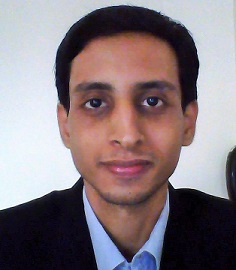
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.