Text inputs are commonly used in HTML forms to accept user input. Many times you may need to allow only alphabet inputs in textboxes of your HTML forms. In this article, we will learn how to allow only alphabet input in HTML text input.
How to Allow only Alphabet Input in HTML Text Input
Here are the steps to allow only alphabet input in HTML text input. Let us say you have the following HTML text input.
<input type='text' id='myText'/>
1. Using HTML
You can directly define the input parsing function as an event listener to onkeydown event.
<input type='text' id='myText' onkeydown="return /[a-z]/i.test(event.key)" />
In the above code, we simply add a return statement to onkeydown event for text input. In the return statement, we use test() function to test the value of event.key that is the key pressed. We test it against a regular expression for alphabets.
In the above example, we have allowed only lowercase letters. If you also want to allow uppercase letters modify the regular expression as shown below.
<input type='text' id='myText' onkeydown="return /[a-zA-Z]/i.test(event.key)" />
2. Using JavaScript
You can also use a JavaScript function to check user input value to see if it is an alphabet or not.
function check_input(event) { var key = event.keyCode; return ((key >= 65 && key <= 90) || key == 8); };
The above function checks the value of entered key to see if it is an alphabet (ASCII – 65 to 90). We have also allowed backspace key (ASCII – 8). You can remove it if you want. Here we have checked only for uppercase alphabets. If you want to check for lowercase alphabets also, then you need to also check if the input value is between ASCII 97 to 122.
function check_input(event) { var key = event.keyCode; return ((key >= 65 && key <= 90) || (key >= 97 && key <= 122) || key == 8); };
You can attach this function to the onkeydown even of the text input.
<input type='text' id='myText' onkeydown="check_input()" />
In this article, we have learnt a couple of simple ways to check if user input in textbox is alphabet. It is good for user validation in HTML forms.
Also read:
How to Allow Only Numeric Input in Textbox
How to Disable Scrolling in HTML/JavaScript
How to Change Sudo Password Timeout in Linux
How to Reset WordPress Admin Password Via MySQL
How to Stop SetInterval Call in JavaScript
Related posts:
Remove Unicode Characters from String
How to Detect Mobile Device Using jQuery
How to Use Variable in Regex in JavaScript
How to Use Dynamic Variable Names in JavaScript
How to Find Element Using XPath in JavaScript
How to Validate Decimal Number in JavaScript
How to Use Variable As Key in JavaScript Object
How to Detect Invalid Date in JavaScript
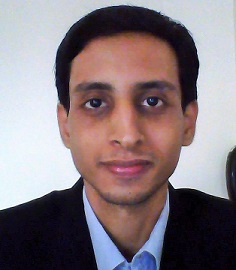
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.