JavaScript is a popular programming language used to develop front end of websites. While using JavaScript, we need to often find elements and perform tasks on them. There are several ways to find DOM elements in JavaScript. You can find them using ID, name attribute, class name, etc. But sometimes you may want to find element using XPath in JavaScript. XPath is typically used to find elements in an XML documents. But they can also be used to find elements in HTML documents. In this article, we will learn how to find element using XPath.
How to Find Element Using XPath in JavaScript
Here are the steps to find element using XPath in JavaScript. We will use document.evaluate() function to find an element using its XPath.
Here is a simple function to do the same.
function getElementByXpath(path) { return document.evaluate(path, document, null, XPathResult.FIRST_ORDERED_NODE_TYPE, null).singleNodeValue; } console.log( getElementByXpath("//html[1]/body[1]/div[1]") );
In the above function, we pass the input parameter XPath to document.evaluate() function as its first argument. We also pass document object to it. We also specify XPathResult.FIRST_ORDERED_NODE_TYPE to return the 1st matching DOM element. singleNodeValue allows you to extract that DOM element.
We call the function on sample XPath that points to the first div on an HTML page.
Alternatively, you can also use XPathEvaluator API to find element using its XPath. It allows you to compile and evaluate XPath expressions.
In the following function, we accept an XPath string as input argument, use it to create an XPath expression. We further call evaluate() function on it to evaluate the expression, and call singleNodeValue to extract the single node.
function getElementByXPath(xpath) { return new XPathEvaluator() .createExpression(xpath) .evaluate(document, XPathResult.FIRST_ORDERED_NODE_TYPE) .singleNodeValue } console.log( getElementByXPath("//html[1]/body[1]/div[1]") );
In this article, we have learnt how to get page DOM element using XPath.
Also read:
How to Remove Submodule in Git
How to Merge Two Git Repositories
How to Setup Git Username & Password for Different Repos
How to Reset Git Credentials
How to Add Images to README.md in GitHub
Related posts:
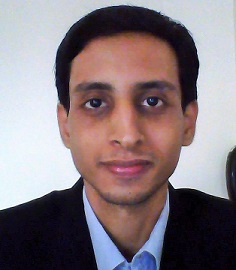
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.