Cookies make it easy for websites to maintain user state information as they browse from one page to another within a domain/subdomain. Often you may need to store or delete information from cookies on your websites, depending on the web page or user actions. You can easily do this using JavaScript or third party libraries like jQuery. In this article, we will learn how to set/unset cookie with jQuery.
How to Set/Unset Cookie With jQuery
Although this article is about setting/unsetting cookie with jQuery, you should know that you don’t really need a third party library for this purpose. Cookies are basically strings that contain variables and their values, which are passed back and forth between client browsers and web server.
Here is the syntax to set cookie via plain JavaScript.
document.cookie="variable=value"
Here is an example to set cookie variable test=hello.
document.cookie="test=hello"
You can set multiple cookie variables by separating them using semi colon (;).
document.cookie="test=hello;location=world"
Once the cookie is set, you can easily retrieve it using the following command. It will return the full cookie string, which you will need to parse into separate variables and values.
var x = document.cookie;
You can delete a cookie variable by setting its value as nothing, as shown below.
document.cookie="test="
If you are already using jQuery on your website, or you need to, then you may need to use jQuery plugins such as this one.
Once you have added the plugin to your website, you can set the cookie with the following command.
$.cookie('name', 'value');
Here is an example to set cookie with the above command.
$.cookie("test", "hello");
You can also set multiple cookie variables in a single command by including a JS object of key-value pairs as shown below.
$.cookie("test", "hello", {expires : 10, domain : 'jquery.com'});
Here is the command to delete the cookie.
$.removeCookie("test");
You can use the following command to read the cookie value.
var cookieValue = $.cookie("test");
In this article, we have learnt how to set/unset cookies using jQuery as well as JavaScript.
Also read:
How to Abort AJAX Request in JavaScript/jQuery
How to Get Value of Text Input Field With JavaScript
How to Merge Two Arrays in JavaScript
How to Persist Variables Between Page Load
How to Scroll to Element With JavaScript
Related posts:
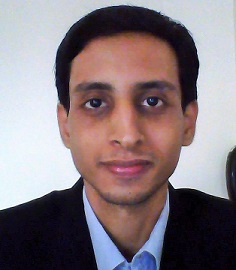
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.