Typically, web developers add event handlers to listen to scrolling event. But sometimes you may need to call functions or features based on scroll direction. In this article, we will learn how to detect scroll direction using JavaScript. There are many third-part libraries for this purpose, but we can easily do this using plain JavaScript.
How to Detect Scroll Direction in JavaScript
Let us say you have a div myDiv whose scrolling direction you need to detect. The first step is to select the element.
var d = document.getElementById("myDiv);
We define a variable to store the position of last scrollTop value and initialize it to zero.
var lastScrollTop = 0;
Next we add an event handler for scroll event. When the user scrolls up or down, the scroll event is frequently called.
element.addEventListener("scroll", function(){ var st = window.pageYOffset || document.documentElement.scrollTop; if (st > lastScrollTop){ // downscroll code } else { // upscroll code } lastScrollTop = st <= 0 ? 0 : st; // For Mobile or negative scrolling }, false);
Every time the event handler for scrolling is called, we retrieve the scrolltop value, compare it with the lastScrollTop value to determine if the scrolling is upwards or downwards. If current scrolltop value is greater than the lastScrollTop value it means user is scrolling down, else scrolling up. We also store the current ScrollTop value as the lastScrollTop value for later use.
Instead of adding the event handler to an element, you can also add it to the entire window if you want, as shown below.
// or window.addEventListener("scroll"....
In this article, we have learn how to detect scroll direction in JavaScript. You can customize it as per your requirement.
It is useful to add customized functionality depending on direction of scrolling for users.
Also read:
How to Split String with Multiple Delimiters in JS
How to Split String With Multiple Delimiters in Python
How to Clone Array in JavaScript
How to Check MySQL Storage Engine Type
How to Use LIKE Operator With Multiple Values
Related posts:
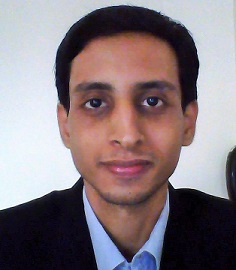
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.