Often web developers need to add time to date values on their websites and applications. There are several ways to do this. You can do it using plan JavaScript or third-party JS libraries. In this article, we will learn how to add 30 minutes to JS date object. You can use these methods to add or subtract other time intervals as well.
How to Add 30 Minutes to JS Date Object
We will learn how to add time to JS date object using JavaScript, as well as third-party libraries.
1. Using JavaScript
Let us say you have the following date object.
var old_time = new Date();
Let us say you want to add 30 minutes to the above date object. You can do using the following command.
diff=30;//time difference in minutes var newDateObj = new Date(old_date.getTime() + diff*60000);
In the above command, we store time difference in diff variable for your understanding. We convert it into milliseconds and add it to the output of getTime() function called on old_date variable. getTime() function returns number of milliseconds in given date object, from Jan 1, 1970. So it is basically adding difference in milliseconds to number of milliseconds in given date object. Then we call Date() constructor to create a date out of the result.
You can also save the above code as a function for easy recall.
function addMinutes(date, minutes) { return new Date(date.getTime() + minutes*60000); }
But ensure that you convert the original date into milliseconds and add the difference in milliseconds. This will take care of things like daylight savings.
2. Using JS library
You can also use JS libraries like Moment.JS or Date.JS for this purpose. Here is a one line code to add 30 minutes to your JS date object.
var new_date = moment(old_date).add(30, 'm').toDate();
In this article, we have learnt how to add 30 minutes to JS Date object. You can also use this method to subtract date difference by using negative numbers.
Also read:
How to Measure Time Taken by JS Function to Execute
How to Replace Part of String in Update Query in MySQL
How to View Live MySQL Queries
How to Generate Random String in MySQL
How to Import Excel File to MySQL
Related posts:
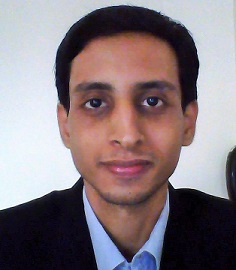
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.