Often web developers need to measure the execution time in JS. It is required to find out which parts of your JS code take the most time, or if there are any bottlenecks. In this article, we will learn how to measure time taken by JS function to execute. There are several ways to do this. We will look at a couple of simple ways that do not require importing any third-party library.
How to Measure Time Taken by JS Function to Execute
Most modern browsers support two APIs – performance and console. You can use either of these web APIs to measure execution time of JS code.
1. Using Performance
performance.now() returns a timestamp when it is called. We will call it twice – before and after our code – to measure start and end time of execution. Then we calculate time taken by calculating the difference between start and end time. Here is an example for your reference.
var startTime = performance.now() doSomething() // <---- code whose execution time needs to be measured var endTime = performance.now() console.log(`Your JS code took ${endTime - startTime} milliseconds`)
Please note, we use back quotes in console.log() function since we need to evaluate the expression calculating the time difference.
You can use this method to measure time for any JS code, not just function calls as we have mentioned above.
If you need to use performance API in NodeJS, you need to import perf_hooks library for it. No imports are required if you are using it on browsers.
const { performance } = require('perf_hooks');
2. Using Console
You can also use console web API to calculate the execution time of JS code. It offers two methods time() and timeEnd() to measure starting and ending time respectively of JS code. Both these methods accept an input string that indicates code block to be tracked.
console.time('test') doSomething() // <---- JS code you're measuring time for console.timeEnd('test')
In the above code, both console.time() and console.timeEnd() accept a string whose value needs to be the same for console API to be able to correctly measure the execution time. If the input string for console.time() and console.timeEnd() are different, then browser won’t be able to measure time properly.
You can also use nesting to measure execution time of multiple code blocks at once.
console.time('test') doSomething() console.time('test2') doSomething() console.timeEnd('test2') doSomething() console.timeEnd('test')
In the above example, we use two console.timeEnd() functions. If we don’t use the correct input string, browser won’t know to which console.time() they correspond to.
In this article, we have seen a couple of simple ways to measure execution time of JS code.
Also read:
How to Replace Part of String in Update Query in MySQL
How to View Live MySQL Queries
How to Generate Random String in MySQL
How to Import Excel File in MySQL
How to View MySQL Log Files
Related posts:
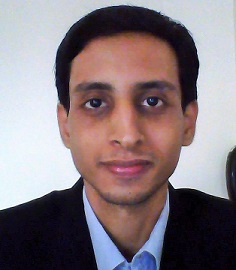
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.