Typically, web developers split JavaScript strings using a single delimiter. But sometimes you may need to split the string using multiple delimiters. In this article, we will learn how to split string with multiple delimiters in JS.
How to Split String With Multiple Delimiters in JS
Let us say you have the following string in JavaScript.
var a = "Hello World,Good Morning";
Let us say you want to split the above string by space as well as comma delimiters.
Generally, developers use split() function to split a string using delimiter. If you want to split the above string by only space, then here is the command to do so.
a.split(' '); ["Hello", "World,Good", "Morning"]
If you want to split the above string by space as well as comma followed by space then you need to use regular expression for this purpose. Regular expressions are enclosed in /…/. In that we, as use [..] to indicate multiple patterns. Within square brackets, we first mention \s to indicate white space character, followed by comma. We also mention + operator to indicate one or more occurrences of any of these characters.
a.split(/[\s,]+/) ["Hello", "World", "Good", "Morning"]
In this article, we have learnt how to split string with multiple delimiters. The key is to provide regular expression in split() function.
Also read:
How to Split String By Multiple Delimiters in Python
How to Clone Array in JavaScript
How to Check MySQL Storage Engine Type
How to Use LIKE Operator for Multiple Values
How to Increase Import File Size in PHPMyAdmin
Related posts:
How to Access Iframe Content With JavaScript
How to Create Please Wait Loading Animation in jQuery
How to Split Array Into Chunks in JavaScript
How to Get Highlighted/Selected Text in JavaScript
How to Allow only Alphabet Input in HTML Text Input
How to Get Image Size & Width Using JavaScript
How to Convert Array to Object in JavaScript
How to Detect Internet Connection is Offline in JavaScript
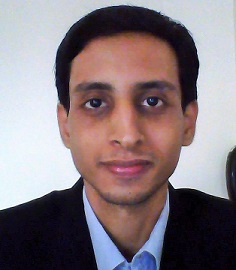
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.