JavaScript arrays are one of the most popular and commonly used to data structures to store and process data in websites. Sometimes you may have a JavaScript array with many elements that you want to split into chunks of equal size, for ease of use. In this article, we will learn how to split array into chunks in JavaScript.
How to Split Array Into Chunks in JavaScript
Here are the steps to split a JavaScript array into chunks. Let us say you have the following array.
array = [1, 2, 3, 4, 5, ...]
We will use slice() function to extract a sub array whose size is equal to the specific chunk size, from our main array. Let us say you want to split array into chunks of array of size 3. We will create a simple for loop that traverses the array and extracts separate chunks from it.
var array = [1, 2, 3, 4, 5, ...]; var chunkSize = 3; for (let i = 0; i < array.length; i += chunkSize) { var chunk = array.slice(i, i + chunkSize); // do something }
For example, the first iteration will extract the first 3 elements of array and create a chunk out of it. In the second iteration, the next 3 items will be extracted, and so on. Please note, depending on size of your array and chunk, the last chunk may be smaller than or equal to chunk size.
Also note, if the chunk size is set to 0, it will result in infinite loop.
The above code will extract sub arrays of chunk size without modifying the original array. If you want to store these chunks in another data structure you can do that as shown below.
var array = [1, 2, 3, 4, 5, ...]; var new_array=[]; var chunkSize = 3; for (let i = 0; i < array.length; i += chunkSize) { var chunk = array.slice(i, i + chunkSize); new_array.push(chunk); }
In this article, we have learnt how to split array into chunks, without modifying the original array in JavaScript. This is very useful if you have a very large array and want to split it into more manageable smaller arrays.
Also read:
How to Print Number With Commas As Thousands
How to Mount Remote Filesystem in Linux
How to Copy Files from Linux to Windows
How to Set Process Priority in Linux
How to Change Default MySQL Data Directory in Linux
Related posts:
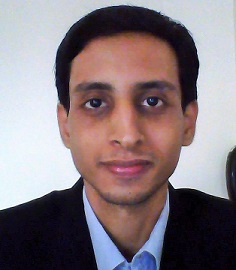
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.