JavaScript objects allow you to store diverse data types in one place as key-value pairs and access them using property keys. They allow you to nest one data inside another resulting in a very compact data storage for large amount of data. Often you may need to clone or copy JavaScript objects. But since they are refences unlike variables, you can copy them using assignment operator ‘=’. There are two types of object cloning available in JavaScript. In this article, we will learn how to deep clone in JavaScript.
How to Deep Clone Objects in JavaScript
Let us first understand the difference between shallow copy and deep copy of JavaScript Objects. JavaScript objects are often nested data structures. Shallow copy means the first level of object is copied and the nested levels are simply referenced. This means that if you change the value of any of the nested data in Shallow copy, it will change the corresponding value in original JS object.
In deep cloning, each and every key-value pair is separately created and saved in the clone object. So if you make changes to a deep clone, it will not affect the original object. There are several ways to do a deep clone of JS Object.
1. Using Structured Cloning
Now there is a standard JS way to deep clone an object using structuredClone constructor available in browsers. This is natively supported in most modern browsers, and will eventually be available in almost all browsers. Here is the syntax for deep copying a JS object using this constructor.
const clone = structuredClone(original);
Please note, this function is available only in modern browsers such as Firefox 94, etc. If it is not available in your browser or your user’s browser, then use the following methods. This will clone all kinds of data such as strings, numbers, dates, functions, etc. properly.
2. Using JSON Library
There is a fast shortcut available to deep clone your JS object if it contains only strings and numbers. Here is the syntax to do it.
const clone = JSON.parse(JSON.stringify(object));
In the above code, JSON library, natively available in almost all browsers, will first convert the JS object into a JSON string and then use it to create a separate JS object that is used as a deep clone. It does not support cloning if your original object contains dates, functions and references. But it is a very useful shortcut that works for most JS objects.
3. Using Lodash.DeepClone
There are many third-party libraries such as Lodash to help you deep clone a JS object. Here is a simple example to use Lodash to do a deep clone of object. It works with all data types including functions and symbols. Here is an example where we clone an array of functions. You just need to mention the JS object name as input parameter for lodash.clonedeep function.
const lodashClonedeep = require('lodash.clonedeep'); const arrOfFunction = [ () => 2, { test: () => 3, }, Symbol('4'), ]; // deepClone copy by refence function and Symbol console.log(lodashClonedeep(arrOfFunction)); // function and symbol are copied by reference in deepClone console.log( lodashClonedeep(arrOfFunction)[0] === lodashClonedeep(arrOfFunction)[0], ); console.log( lodashClonedeep(arrOfFunction)[2] === lodashClonedeep(arrOfFunction)[2], );
In this article, we have learnt how to deep clone JS objects in JavaScript. If structured cloning is supported by your web browser use it. Else, if your object contains only numbers and strings, you can use JSON.
Also read:
How to Include JavaScript File in Another JavaScript File
How to Add Event to Dynamic Elements in JavaScript
How to Clone Objects in JavaScript
How to Replace All Occurrences of String in JavaScript
Python String Slicing
Related posts:
How to Attach Event to Dynamic Elements in JavaScript
How to Add Days to Date in JavaScript
How to Get Length of JavaScript Object
How to Get Random Element from Array in JavaScript
How to Detect Click Outside Element in JavaScript
How to Pass Parameter to SetTimeout Callback
How to Scroll to Bottom of Div
How to Remove Key from JavaScript Object
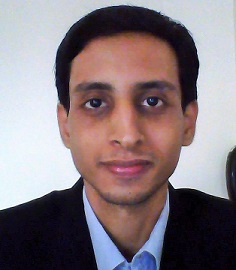
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.