JavaScript allows you to easily manipulate strings as per your requirement. You can split strings, concatenate them and even replace one or more characters in them. Sometimes you may need to replace all occurrences of string in JavaScript. Typically, developers use replace() function that only replaces the first occurrence of string or character. By slightly modifying its input values, you can get it to replace all occurrences of string. In this article, we will learn a couple of ways to do this easily.
How to Replace All Occurrences of String in JavaScript
Typically, developers use replace() function to replace substring in JavaScript. Here is its syntax.
string.replace(old_substring, new_substring)
Here is an example to replace substring abc with pqr.
string='abc def abc'; console.log(string.replace('abc','pqr');
You will see the following output.
pqr def abc
As you can see above, the replace() function only replaces the first occurrence of substring in JavaScript. If you need to replace all occurrences of string in JavaScript, you will need to enclose the string to be replaced within /…/g.
Here is an example to replace all occurrences of ‘abc’ in above mentioned string with pqr.
string='abc def abc'; console.log(string.replace(/abc/g,'pqr');
You will see the following output.
pqr def pqr
In the above case /abc/g is treated as a regular expression and all substrings that match the regular expression are replaced. Please note, mention the string to be replaced without quotes.
You can also use other regular expressions within /…/g to determine other substrings. Here is an example to replace all occurrences of numbers in your string.
str='ernen22bb3kb3b43'; console.log(str.replace(/[0-9]/g,'');
You will see the following output.
ernenbbkbb
Alternatively, you can also use regular expression shortcuts such as \d for digits, in replace function. Here is the above example using regex shortcut.
str='ernen22bb3kb3b43'; console.log(str.replace(/\d/g,'');
The above code will also give you the same output.
ernenbbkbb
Sometimes the string you want to replace may be available as a variable. In this case, you need to create a regular expression out of the substring before you specify it in replace() function. Here is an example for it.
var str='abc def abc'; var find = 'abc'; var re = new RegExp(find, 'g'); str = str.replace(re, 'pqr'); console.log(str);
You will see the following output.
pqr def pqr
This is very useful in case the string you want to replace is present as a variable, and you want to replace all occurrences of this string.
Alternatively, in the latest browsers, you can also use replaceAll() function to replace all occurrences of string. Here is an example.
string='abc def abc'; console.log(string.replaceAll('abc','pqr');
You will see the following output.
pqr def pqr
In this case, you need to mention the string to be replaced ‘abc’ within quotes.
In this article, we have learnt several ways to replace all occurrences of string in JavaScript. You can use it to replace one or more characters in a string in JavaScript. You can use this on almost every browser.
Also read:
How to Select Rows from Dataframe Using Column Values
How to Rename Columns in Pandas
How to Create Pandas Dataframe from Dictionary
How to Create Pandas Dataframe from Lists
How to Access Index of Last Element in Pandas Dataframe
Related posts:
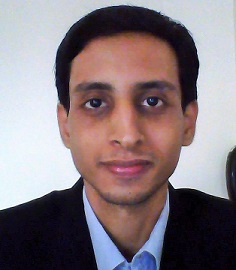
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.