Sometimes you may need to take screenshot of Div in JavaScript in your website or web application. There are many JavaScript libraries for this purpose. For our tutorial, we will be using html2canvas library. You can download it from here. Taking screenshot is very useful for debugging and testing purposes. You can simply add a button to your website or application to allow users to take a screenshot and send them to you.
How to Take Screenshot of Div in JavaScript
Here are the steps to take screenshot of div in HTML.
1. Create HTML Document
First create a blank HTML document. Include html2canvas library from downloaded file or link to CDN, highlighted in bold below..
<!DOCTYPE html> <html> <head> <title> How to take screenshot of a div using JavaScript? </title> <!-- Include from the CDN --> <script src= "https://cdn.jsdelivr.net/npm/html2canvas@1.0.0-rc.5/dist/html2canvas.min.js"> </script> <!-- Include locally otherwise --> <!-- <script src='html2canvas.js'></script> --> </head> <body> </body> </html>
2. Create Div
In HTML body, create div element whose screenshot you need to take, and also another div, where you will save the output.
<div id="photo"> <h1>Test Screenshot</h1> Hello everyone! This is a trial page for taking a screenshot. <br><br> This is a dummy button! Click the button to take a screenshot of the div. <br><br> </div> <h1>Screenshot:</h1> <div id="output"></div>
The idea is to take the screenshot of first div with the click of a button, and store its result in second div. We will add the button in next step.
3. Add Button
Next, create a button inside the div which when clicked will take screenshot. We also add an onclick() handler for the button.
<button onclick="takeshot()"> Take Screenshot </button>
When you click the above button, it will call function takeshot(), which we define in next step using JavaScript.
4. Add Click Handler
Lastly, we add a onclick handler for button, which will take screenshot, when you click the button. It will use html2canvas library and append the body to the page. We will add it within <script> tag since it is JavaScript function.
<script type="text/javascript"> // Define the function // to screenshot the div function takeshot() { let div = document.getElementById('photo'); // Use the html2canvas // function to take a screenshot // and append it // to the output div html2canvas(div).then( function (canvas) { document .getElementById('output') .appendChild(canvas); }) } </script>
In the above JavaScript code, we first get hold of our first div using its ID=’photo’ attribute. Then we pass this object to html2canvas, which appends it to div with ID=’output’. Of course, you can do whatever you want with this screenshot, instead of appending it to another div.
Here is the entire code for your reference.
<!DOCTYPE html> <html> <head> <title> How to take screenshot of a div using JavaScript? </title> <!-- Include from the CDN --> <script src= "https://cdn.jsdelivr.net/npm/html2canvas@1.0.0-rc.5/dist/html2canvas.min.js"> </script> <!-- Include locally otherwise --> <!-- <script src='html2canvas.js'></script> --> </head> <body> <div id="photo"> <h1>Test Screenshot</h1> Hello everyone! This is a trial page for taking a screenshot. <br><br> This is a dummy button! Click the button to take a screenshot of the div. <button onclick="takeshot()"> Take Screenshot </button> <br><br> </div> <h1>Screenshot:</h1> <div id="output"></div> <script type="text/javascript"> // Define the function // to screenshot the div function takeshot() { let div = document.getElementById('photo'); // Use the html2canvas // function to take a screenshot // and append it // to the output div html2canvas(div).then( function (canvas) { document .getElementById('output') .appendChild(canvas); }) } </script> </body> </html>
When you click the button, the click handler will take a screenshot and save it to second div with id=’output’. After that happens, you can right click the screenshot and save it as image for future reference.
In this article, we have learnt how to take screenshot of div in HTML. You can customize the code to take screenshot of most HTML elements by their ID.
Also read:
How to Create Incremental Backup in Linux
How to Improve Ubuntu Speed & Performance
How to Embed PDF in HTML
How to Run Multiple Python Files At Once
How to Merge PDF Files Using Python
Related posts:
How to Find Last Occurrence of Character in String in Javascript
How to Change Element's Class in JavaScript
How to Take Screenshot in Ubuntu Terminal
How to Restore MongoDB Dump in Windows & Linux
How to Backup WordPress to Dropbox
How to Include Another HTML in HTML File
VPS vs Shared Hosting : In-Depth Comparison
How to Write Limit Query in MongoDB
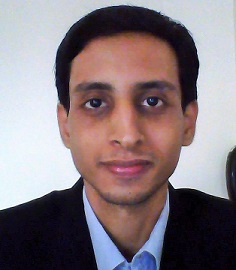
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.