Sometimes you may need to check if variable, string or object is number in Python. Here are the steps to check if variable in number in Python. There are several ways to do this in python. We will look at each of them one by one.
How to Check if Variable is Number in Python
Python has dynamic type setting for a variable, that is, a python variable’s type depends on the value it stores, and is evaluated during runtime. Here is an example where we assign a number to python variable, and then re-assign it as string.
>>> a=1 >>> print a 1 >>> a='abc' >>> print a 'abc'
Using Type
But sometimes you may need to determine the type of a variable in order to process it, or process only specific types of variables. In such cases, you can easily use type() function to determine if a variable, string, or even object is number or not.
>>> a = 1 >>> print(type(a)) <type 'int'> >>> a = 1.0 >>> print(type(a)) <type 'float'> >>> a = 's' >>> print(type(a)) <type 'str'>
Now if you want to check if a variable is number or not, here is the command for it.
if type(a) == int or type(a == float: #do something
Please note, when you check if a variable is int or float, you need to mention these keywords in the if condition, without using any quotes. Otherwise, you will get an error.
Using Numbers
Alternatively, you can also check if a variable is number using number module.
>>> import numbers >>> variable = 5 >>> print(isinstance(5, numbers.Number)) True
Using try…except
You can also use try..except block to determine if a variable is int or float. For example, in our try block, we will cast our variable to int. If the variable is indeed an int, it will no give an error, else it will raise an exception.
a = 1 try: tmp = int(a) print('The variable a number') except: print('The variable is not a number')
Please note, the try block will work even if you try to convert int to float and vice versa. In this article, we have learnt how to check if variable, string or object is number or not.
Also read:
How to Take Backup of MySQL Database in Python
How to Prevent Accidental File Deletion in Linux
How to Make File & Directory Undeletable in Linux
How to Disable Auto Logout in Linux
How to Make File Unreadable in Linux
Related posts:
How to Import Other Python File
How to Drop One or More Columns in Python Pandas
How to Copy List in Python
How to Concatenate Items in List Into String in Python
How to Store JSON to File in Python
How to Configure Python Flask to be Externally Visible
Python Script to Run SQL Query
How to Create Python Function with Optional Arguments
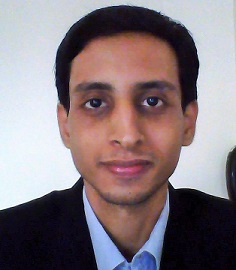
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.