Sometimes you may need to test if a certain variable is number or not in shell script, and process code depending on its data type. In this article, we will learn how to test if variable is number in shell script. You can use these steps in almost every Linux distribution.
How to Test If Variable is Number in Shell Script
There are several ways to check if variable is number in shell script.
1. Regular Expression
The simplest way is to check variable against regular expression for numbers. Here is a simple shell script to check if your variable is an integer.
re='^[0-9]+$' if ! [[ $yournumber =~ $re ]] ; then echo "error: Not a number" >&2; exit 1 fi
The first statement defines a regular expression for integers. Then we check the variable $yournumber against the regular expression $re and echo message if it is not a regular expression. The following condition will evaluate to false for non-numbers.
$yournumber =~ $re
If your variable is not an integer but a floating point number or decimal, with point(.) in between, then you can modify the regular expression.
re=^[0-9]+([.][0-9]+)?$
Similarly, if your variable also contains signs +/-, then you can further modify your regular expression to handle them.
re=^[+-]?[0-9]+([.][0-9]+)?$
You can also employ a switch case statement to check your variable against the regular expression.
case $string in ''|*[!0-9]*) echo 'not number' ;; *) echo 'number' ;; esac
2. Using Equality Operators
If you are using basic shells such as Bourne shells, then you can use equality operator on the variable to check if it is a number or not. This is because, if you use numerical operators on non-numerical variables, you will get an error. Here is a simple example to demonstrate this.
#!/bin/bash var=a if [ -n "$var" ] && [ "$var" -eq "$var" ] 2>/dev/null; then echo number else echo not a number fi
In the above code, we check if variable $var is not null and then we use the following condition which works on only numerical operators.
"$var" -eq "$var"
But please note, this works only with integers and not numbers with decimal point.
In this article, we have learnt a couple of simple ways to determine if a shell variable is number or not. Using regular expressions is a comprehensive way to check if variable is numeric or not.
Also read:
How to Check if Number is Variable in Python
How to Take Backup of MySQL Database in Python
How to Prevent Accidental File Deletion
How to Make File & Directory Undeletable in Linux
How to Disable Auto Logout in Unix
Related posts:
How to Unzip File in Linux
How to Set or Change Hostname in CentOS / RHEL
How to Stop Linux Package Update in Ubuntu
How to Know Which Shell I am Using in Linux
How to Find & Replace String in VI Editor
How to List All Cron Jobs for All Users
How to Check if Port is Open or Closed in Linux
How to Make File and Directory Undeletable in Linux
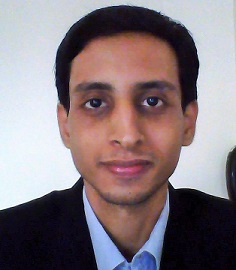
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.