Python is a popular language that offers several data structures to store data. Lists are powerful python data structures that allow you to copy diverse data types in one place in a compact manner. They also offer many out-of-the-box functions. Sometimes you may need to copy lists in python. In this article, we will learn how to copy list in python.
How to Copy List in Python
In python, there are two ways to copy lists – deep copy and shallow copy. In deep copy, python will create an entirely separate list object that is not connected in source list in anyway, so when you make changes in one of them, the other is not affected. In shallow copy, the new list consists of pointers to the old list. So when you make changes in new list, the old list also changes. We will look at both ways to copy lists in python.
1. How to Shallow Copy List in Python
You can shallow copy using the default copy() function available for each list. We will copy the list and then change one element in our old list to see what happens to the new list.
>>> a = [[1, 2, 3], [4, 5, 6]] >>> a [[1, 2, 3], [4, 5, 6]] >>> b=list(a) >>> b [[1, 2, 3], [4, 5, 6]] >>> a[0][1] = 11 >>> a [[1, 11, 3], [4, 5, 6]] >>> b [[1, 11, 3], [4, 5, 6]]
In this case, when we change the old list after making a copy, it changes the new list also.
2. How to Deep Copy List in Python
You can deep copy list using deepcopy function. Here also we will copy the list and then change one element in our old list to see what happens to the new list.
>>> a = [[1, 2, 3], [4, 5, 6]] >>> a [[1, 2, 3], [4, 5, 6]] >>> import copy >>> a=[1,2,3] >>> b=copy.deepcopy(a) >>> b [[1, 2, 3], [4, 5, 6]] >>> a[0][1] = 11 >>> a [[1, 11, 3], [4, 5, 6]] >>> b [[1, 2, 3], [4, 5, 6]]
In this case, when you we change the old list, the new list does not change.
In this article, we have learnt how to copy lists in python.
Also read:
How to Copy Files in Python
How to Comment in Python
Git Rename Local & Remote Branch
How to Create Remote Git Branch
How to Unstage Files in Git
Related posts:
How to Run Python Script in Apache Web Server
How to POST JSON Data in Python Requests
How to Convert PDF to CSV in Python
How to Check if Key Exists in Python Dictionary
How to Check if File Exists in Python
How to Drop One or More Columns in Python Pandas
How to Create Nested Directory in Python
How to Check if Variable is Number in Python
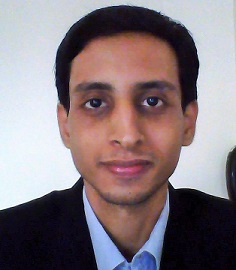
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.