Python dictionary is a powerful data structure that allows you to easily store different data types as key-value pairs. But if you try to access a dictionary value of a key that does not exist, you will get an error and your python code might stop running. So it is advisable to check if a key exists before referring to it in python. In this article, we will learn how to check if key exists in Python dictionary. There are several ways to do this and we will look at some simple ones.
How to Check if Key Exists in Python Dictionary
Let us say you have the following dictionary.
d = {"k1": 100, "k2": 200}
Let us say you try getting value of key k3 which does not exist in above dictionary.
>>> d['k3'] Traceback (most recent call last): File "<pyshell#1>", line 1, in <module> d['k3'] KeyError: 'k3'
1. Using In Operator
One of the simplest ways to check if a key exists in python dictionary is to use in operator. Here is how you can use it to check if key k3 exists in your dictionary.
if "k1" in d: print('key is present') if "k3" in d: print("key is not present")
In the above code, we check if key k1 is present and then if key k3 is present and display messages accordingly.
2. Using get() function
You can also use get() function to assign default value in case the key does not exist. Here is an example.
f = d.get('k3',0) print(f) output: 0
In the above code, since key k3 does not exist in the dictionary, it assigns the default value 0 to variable f.
3. Using setdefault() function
setdefault() function is similar to get() function. It checks if the key exists, else returns the default value.
>>> d.setdefault('k3',1) 1
In the above code, since key k3 does not exist in the dictionary, it returns default value 1.
In this article, we have learnt several simple ways to check if a key exists in a dictionary or not.
Also read:
NGINX Catch All Location
How to Download Images in Python
How to Remove Trailing Newline in Python
How to Pad String in Python
How to Get Size of Object in Python
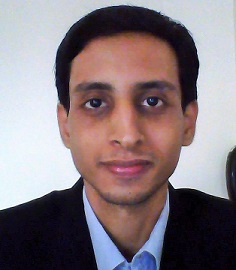
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.