Typically, people use commands like mv or cp in Linux, and move command in Windows to move files around your system. Sometimes you may need to move file from within your python script as a part of your website or application. There are several python modules available for this purpose. In this article, we will learn how to move file in Python.
How to Move File in Python
Let us say you have a file at /home/ubuntu/data.txt and you want to move it to /home/projects/data.txt
You can easily do this using os module as shown below. It provides os.rename() and os.replace() utilities for this purpose.
import os os.rename("/home/ubuntu/data.txt", "/home/projects/data.txt") os.replace("/home/ubuntu/data.txt", "/home/projects/data.txt")
You can also do the same thing using shutil module.
import shutil shutil.move("/home/ubuntu/data.txt", "/home/projects/data.txt")
All the above commands have the same syntax where the 1st argument is the source file path and 2nd argument is the destination file path.
In all the above commands you must mention the same file name if you want to move it. If you don’t mention the file name or mention a different filename in 2nd argument, then the file be renamed as well as moved.
Also, in case of os.rename() and os.replace() the destination folder must exist, otherwise they will give an error.
While using os.rename() on Windows, the destination file must not already exist, else it will throw an exception. But os.replace() command will silently replace the file in this case.
shutil.move() function basically calls os.rename() function in most cases. shutil.move() works on different disks and devices but os.rename() and os.replace() won’t work in these cases. Also you can use shutil.move() to move multiple files in a folder, or even folders. If the destination is on a different disk, it will copy and delete the original file.
Starting python 3.4+, you can also use pathlib to move file in Python.
from pathlib import Path Path("/home/ubuntu/data.txt").rename("/home/projects/data.txt")
In this article, we have learnt how to move files in Python.
Also read:
How to Reset Auto Increment in MySQL
How to Show Last Queries Executed in MySQL
How to Change Href Attribute of Link Using jQuery
How to Get URL Parameters Using JavaScript
How to Convert String to Date in MySQL
Related posts:
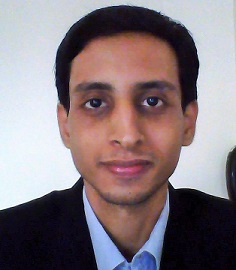
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.