Python dictionaries are useful data structures that allow you to store diverse data types in a compact manner. Sometimes you may need to get union of two dictionaries in Python. In this article, we will look at different ways to merge two dictionaries in Python.
How to Merge Two Dictionaries in Python
There are multiple ways to combine two dictionaries in Python. We will look at each of them.
Union of dictionaries in Python 2.x
If you run python 2.x version, then you can merge two dictionaries using update() function. Here is an example,
>>> a={1:'1',2:'2'} >>> b={2:'2',3:'3'} >>> a.update(b) >>> a {1: '1', 2: '2', 3: '3'}
Please note, update() function can only be used to merge one dictionary into another. It does not create a third dictionary, and returns None.
Also read : How to View Hidden Files in Linux
Union of Dictionaries in Python 3.x
If you use Python 3.9 or higher, you can simply use the | operator to merge two dictionaries in python
>>> a={1:'1',2:'2'} >>> b={2:'2',3:'3'} >>> c= a | b >>> c {1: '1', 2: '2', 3: '3'}
In python 3.5 or higher, you can even use ** operator to merge two dictionaries as shown.
>>> a={1:'1',2:'2'} >>> b={2:'2',3:'3'} >>> c = {**a, **b} >>> c {1: '1', 2: '2', 3: '3'}
Unlike update() function, the above operators result in creation of third dictionary, leaving the two dictionaries unchanged.
That’s it. As you can see, it is very easy to merge two dictionaries in python.
Also read :
How to Get Difference Between Two Python Dictionaries
How to Intersect Two Python Dictionaries
Related posts:
How to Convert Bytes to String in Python
How to Shuffle List of Objects in Python
How to Remove Punctuation from String in Python
How to Concatenate Multiple Lists in Python
How to Compare Dictionary in Python
How to Lock File in Python
How to List All Files in Directory in Python
How to Raise Exception in Python
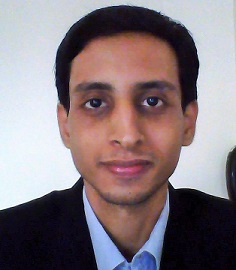
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.