Sometimes you may need to list all files & subdirectories in a specific directory. Python provides several useful libraries & modules that automatically traverse your folder and output a list of files & subfolders present in them. In this article, we will look at how to list all files in directory in Python.
How to List All Files in Directory in Python
Python allows you to traverse the folder structure using os.listdir, os.walk, glob and more. Here are the different ways to list all files in directory. Let us say you want to list all files & subfolders present in /home/ubuntu.
1. Using os.listdir
os.listdir() method gives you the list of all files & directories in a specified path. By default, it does so for your present working directory.
Here is the syntax.
os.listdir(path)
Here is a simple example.
# import OS module import os # Get the list of all files and directories path = "/home/ubuntu" dir_list = os.listdir(path) print("Files and directories in '", path, "' :") # prints all files print(dir_list) Output Files and directories in /home/ubuntu ['data.txt','/project/','file.jpg']
If you only want to get list of txt files or files with a specific extension, you can use the endswith function as shown below.
#import OS import os path = "/home/ubuntu" for x in os.listdir(): if x.endswith(".txt"): # Prints only text files print(x)
2. Using os.walk()
You may also use os.walk() method to traverse a folder’s contents. It will yield two lists for each directory it visits – one for files and the other for folders.
# import OS module import os # This is my path path="/home/ubuntu" for (root, dirs, files) in os.walk(path): for f in files: print(f)
In the above example, you get 2 lists – files and dirs. You can loop through the files list to display filenames, and through dirs loop through to display folders. In the above example, we loop through files list to display only filenames.
If you only want to list .txt files add an if condition as shown below in bold.
# import OS module import os # This is my path path="/home/ubuntu" for (root, dirs, files) in os.walk(path): for f in files: if '.txt' in f: print(f)
3. Using os.scandir()
os.scandir() is available only for python 3.5 and above. Here is its syntax.
os.scandir(path = /path/to/folder)
It returns an iterator. Here is an example.
# import OS module import os # This is my path path="/home/ubuntu" obj = os.scandir() # List all files and directories in the specified path print("Files and Directories in '% s':" % path) for entry in obj: if entry.is_dir() or entry.is_file(): print(entry.name) Output file.txt data.jpg export.csv
4. Using glob
You may also use glob() method to get list of files & folders in a specific path pattern. glob allows you to use wildcard expressions in your path allowing you to traverse multiple folders at once. Here is an example.
import glob # This is my path path="/home/ubuntu" # Using '*' pattern print('\nList of files:') for files in glob.glob(path + '*'): print(files) Output List of files: data.txt file1.csv export.txt
You may also use iglob method to recursively print file and folder names. Here is its syntax.
glob.iglob(pathname, *, recursive=False)
Here is an example.
import glob path="/home/ubuntu" for file in glob.iglob(path, recursive=True): print(file)
In this article, we have looked at several different ways to traverse a folder and list its file and subdirectories. Of these os.listdir() is most commonly used. Most of these methods return a list, while some return an iterator that you can loop over to display file & directory names as per your requirement.
Also read:
How to Send HTML Email With Attachment in Python
Postfix Mail Server Configuration Step by Step
How to Install GoAccess Log Analyzer in Ubuntu
How to Check Python Package Version
How to Install Go (Golang) in Ubuntu
Related posts:
Plot Graph from CSV Data Using Python Matplotlib
How to Execute Stored Procedure in Python
How to Extract Tables from PDF in Python
How to Read Binary File in Python
What Is __name__ In Python
How to Merge Folders & Directories in Python
Random Password Generator in Python with Source Code
How to Use Decimal Step Value for Range in Python
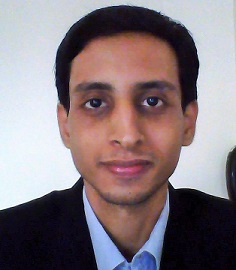
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.