Sometimes you may need to plot CSV data into graphs and charts. This can be quite tedious if you are trying to do this programmatically. Python provides some excellent libraries to easily plot CSV data into graphs and charts. You can use them to quickly visualize CSV data, as a standalone task, or even within your application/website. In this article, we will learn how to plot graph CSV data using python matplotlib library.
Plot Graph from CSV Data Using Python Matplotlib
For our article, we will use two python libraries – pandas and matplotlib. Python pandas is a superb library that allows you to easily work with data. You can use it to load data from files to python objects that work like tables, run tasks on columns and rows, combine or split tables, export data to different file formats, and do a lot more.
Matplotlib is a popular python library that allows you to easily plot graphs & charts from data. It provides an object-oriented API for plotting data as well as embedding them into applications.
First, we will install these two libraries, since they are not available in python by default. Open terminal and run the following command to install them.
$ pip install matplotlib $ pip install pandas
Run the following command to create a new python file.
$ vi plot_csv.py
Add the following lines to it.
#!/usr/bin/env python import pandas as pd import matplotlib.pyplot as plt
The above lines sets the execution environment and imports pandas & matplot library.
Next, we set the size of plot area, and also set the padding to autoadjust.
plt.rcParams["figure.figsize"] = [7.50, 3.50] plt.rcParams["figure.autolayout"] = True
Next, we make a list of headers to be read from our CSV file.
headers = ['Name', 'Age', 'Marks']
Next, we read the CSV file with headers. We will use the read_csv() function to easily do this. It will return a python dataframe object that we will use to plot graph. We will also pass the above defined headers list as names argument.
df = pd.read_csv('marks.csv', names=headers)
Please note, if your CSV file is not in the same folder as your python file, you need to provide the full path to csv file, as the first argument of read_csv function.
We set the index, or column to be plotted on the X-axis using set_index() function. We also call the function plot(), chained to set_index(). This will plot the data with the Name column used as X-axis and each of the other columns (age,marks) used as separate Y-axis series.
df.set_index('Name').plot()
Finally, we call show() function on our matplotlib object to display the graph.
plt.show()
Here is the complete code for your reference.
#!/usr/bin/env python import pandas as pd import matplotlib.pyplot as plt plt.rcParams["figure.figsize"] = [7.50, 3.50] plt.rcParams["figure.autolayout"] = True headers = ['Name', 'Age', 'Marks'] df = pd.read_csv('marks.csv', names=headers) df.set_index('Name').plot() plt.show()
Make the file executable.
$ sudo chmod plot_csv.py
Now you can run the file with the following command to plot your CSV data.
$ python plot_csv.py
In this article, we have learnt how to plot graph from CSV data. You can customize it as per your requirement. Pandas library is great for data analytics and processing. Matplotlib is useful for graphing and data visualization. Using pandas and matplotlib, you can easily plot CSV data to graphs, and use it in your application/website.
Also read:
How to Encrypt Password in Python
How to Create Thumbnail from Image in Python
How to Open Multiple Files in Vim
How to Split Vim Screen Horizontally & Vertically
Fix Unable to Mount NTFS Error in Linux
Related posts:
How to Get Filename from Path in Python
How to Use Decimal Step Value for Range in Python
How to Check if Substring is in List of Strings
How to Fix NoReverseMatch Error in Django
How to Setup Uwsgi with NGINX for Python
How to Count Repeated Characters in String in Python
Python Script to Run SQL Query
How to Check if String Matches Regular Expression
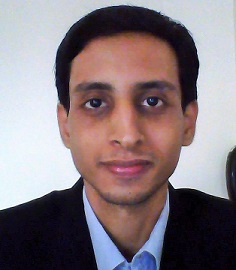
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.